Introduction to PowerShell for Cybersecurity
Leveraging Automation for Enhanced Security
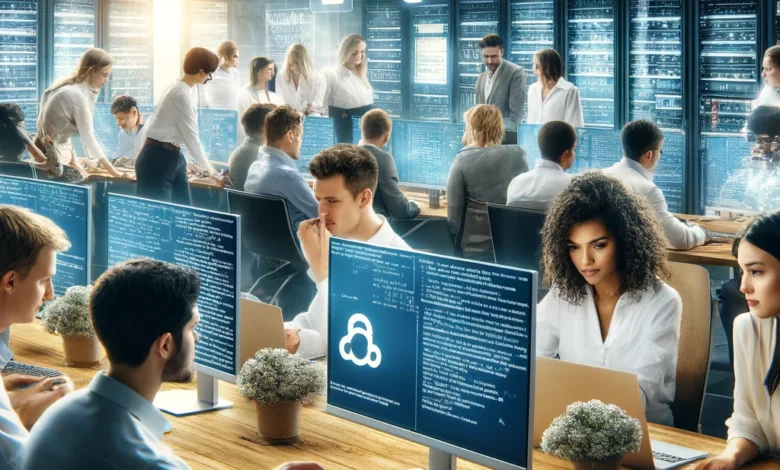
Today, we’re diving into the powerful world of PowerShell—a versatile scripting language and command-line shell developed by Microsoft. In the realm of cybersecurity, PowerShell stands out as an indispensable tool, offering unparalleled capabilities for automating tasks, managing systems, and enhancing security operations.
PowerShell is more than just a command-line interface; it’s a comprehensive scripting environment that allows cybersecurity professionals to streamline their workflows, increase efficiency, and respond more effectively to threats. PowerShell has become a cornerstone of modern cybersecurity practices with its extensive library of built-in commands, known as cmdlets, and its ability to integrate with other technologies.
Here, we’ll provide you with an introduction to PowerShell, highlighting its significance in cybersecurity and the many benefits it offers. You’ll learn about its basic syntax, some common cmdlets, and how PowerShell can be leveraged to automate repetitive tasks and improve security measures.
By the end of this guide, you’ll have a solid foundation in PowerShell and a clear understanding of why it’s an essential tool for any cybersecurity professional. So, let’s embark on this journey together and unlock the potential of PowerShell to elevate your cybersecurity game.
Understanding PowerShell: Basics and Syntax
PowerShell is a powerful scripting language that combines the functionality of a command-line shell with the flexibility of a scripting language. Understanding its basics and syntax is essential for leveraging its full potential in cybersecurity tasks.
Basic Elements of PowerShell
Cmdlets:
Definition: Cmdlets are lightweight commands used in the PowerShell environment. They follow a Verb-Noun naming pattern, making it easy to understand and use.
Examples:
Get-Process
: Retrieves information about running processes.
Set-ExecutionPolicy
: Changes the user preference for the PowerShell script execution policy.
Syntax Rules:
Commands: PowerShell commands are case-insensitive and can be written in a straightforward manner. For example:
Parameters: Cmdlets often have parameters that modify their behavior. Parameters are prefixed with a dash (-
), such as -Name
or -Force
.
Mathematica code
Get-Help Get-Process
Mathematica code Get-Service -Name "wuauserv"
Pipelines: PowerShell uses pipelines (|
) to pass the output of one cmdlet as input to another, enabling powerful command chaining.
vbnet code
Get-Process | Where-Object { $_.CPU -gt 100 }
Script Structure:
Variables: Variables in PowerShell are declared using the dollar sign ($
), and they store data that can be used and manipulated.bashCopy code$username = "Admin"
Loops and Conditional Statements: PowerShell supports loops (for
, foreach
, while
) and conditional statements (if
, else
, switch
) for control flow.
Powershell code
foreach ($process in Get-Process) { if ($process.CPU -gt 100) { Write-Output "$($process.Name) is using high CPU" } }
Functions: Functions allow you to encapsulate code into reusable blocks. They are defined using the function
keyword.
powershell code
function Get-HighCPUProcesses { Get-Process | Where-Object { $_.CPU -gt 100 } }
Simple Script Examples
Hello World:
A classic example to illustrate the basic script structure.
powershell code
Write-Output "Hello, World!"
Listing Services:
A script to list all running services on a system.
powershell code
Get-Service | Where-Object { $_.Status -eq 'Running' }
Checking Disk Space:
A script to check the free disk space on all drives.
powershell code
Get-PSDrive -PSProvider FileSystem | Select-Object Name, @{Name="FreeSpaceGB";Expression={[math]::round($_.Free/1GB,2)
}
}
By grasping these basic elements and syntax rules, you can start writing your own PowerShell scripts to automate tasks and manage systems effectively. In the next section, we’ll delve into essential cmdlets for cybersecurity, showing you how to harness the power of PowerShell in your security operations.
Essential Cmdlets for Cybersecurity
PowerShell is equipped with a vast array of cmdlets that are particularly useful for various cybersecurity operations. These cmdlets help in gathering system information, configuring networks, and managing security settings. Below, we explore some of the essential cmdlets for cybersecurity and provide practical examples of their use.
System Information Cmdlets
Get-Process:
Description: Retrieves information about the processes running on a computer.
Example:
powershell code
Get-Process | Where-Object { $_.CPU -gt 100 }
This command lists all processes consuming more than 100 units of CPU.
Get-Service:
Description: Retrieves the status of services on a local or remote machine.
Example: powershell code
Get-Service | Where-Object { $_.Status -eq 'Running' }
This command lists all running services on the system.
Get-WmiObject:
Description: Retrieves management information from local and remote computers.
Example: powershell code
Get-WmiObject -Class Win32_ComputerSystem
This command provides detailed information about the computer system.
Network Configuration Cmdlets
Get-NetIPAddress:
Description: Retrieves IP address configuration.
Example: powershell code
Get-NetIPAddress
This command displays the IP address configuration for all network adapters.
Test-Connection:
Description: Sends ICMP echo request packets (pings) to one or more computers.
Example: powershell code
Test-Connection -ComputerName google.com -Count 4
This command pings Google four times to test connectivity.
Get-NetFirewallRule:
Description: Retrieves the firewall rules on the local or remote computer.
Example: powershell code
Get-NetFirewallRule | Where-Object { $_.Enabled -eq $true }
This command lists all enabled firewall rules.
Security Management Cmdlets
Get-LocalUser:
Description: Retrieves local user accounts.
Example: powershell code
Get-LocalUser
This command lists all local user accounts on the computer.
Set-ExecutionPolicy:
Description: Changes the user preference for the PowerShell script execution policy.
Example: powershell code
Set-ExecutionPolicy -ExecutionPolicy RemoteSigned
This command sets the execution policy to allow scripts signed by a trusted publisher.
Get-EventLog:
Description: Retrieves the events from event logs on the local or remote computers.
Example:powershell code
Get-EventLog -LogName Security -Newest 10
This command retrieves the 10 most recent entries from the Security event log.
Practical Examples
Auditing User Logins:
Objective: To monitor user logins and identify any unauthorized access attempts.
Cmdlet: powershell code
Get-EventLog -LogName Security -InstanceId 4624 | Select-Object TimeGenerated, ReplacementStrings
This command retrieves successful login events.
Checking Installed Updates:
Objective: To ensure the system is up to date with security patches.
Cmdlet: powershell code
Get-HotFix
This command lists all installed updates and hotfixes.
Monitoring Network Connections:
Objective: To identify suspicious network connections.
Cmdlet: powershell code
Get-NetTCPConnection | Where-Object { $_.State -eq 'Established' }
This command lists all established TCP connections.
By incorporating these essential cmdlets into your PowerShell scripts, you can streamline and enhance your cybersecurity operations. Next, we’ll explore how to automate tasks using PowerShell, which can significantly improve your efficiency and effectiveness in managing security tasks.
Automating Tasks with PowerShell
PowerShell’s scripting capabilities make it an invaluable tool for automating repetitive tasks, which not only saves time but also reduces the likelihood of human error. By automating common cybersecurity tasks such as log analysis, user account management, and system monitoring, you can focus on more complex and strategic activities. Below, we detail how to write scripts for these tasks and highlight their benefits.
Automating Log Analysis
Log analysis is a critical task in cybersecurity, helping to identify potential security incidents and compliance issues. Automating this process ensures consistent and timely analysis.
Script to Extract Failed Login Attempts: powershell code
$failedLogins = Get-EventLog -LogName Security -InstanceId 4625 $failedLogins | Select-Object TimeGenerated, EntryType, InstanceId, Message | Export-Csv -Path "C:\Logs\FailedLogins.csv" -NoTypeInformation
Script to Monitor System Events: powershell code
$events = Get-EventLog -LogName System -Newest 100 $criticalEvents = $events | Where-Object { $_.EntryType -eq "Error" } $criticalEvents | Select-Object TimeGenerated, EntryType, Source, Message | Export-Csv -Path "C:\Logs\SystemErrors.csv" -NoTypeInformation
Quickly identifies and records critical system errors.
Helps in proactive system maintenance and issue resolution.
Automatically generates a report of failed login attempts, which can be reviewed for suspicious activity.
Ensures logs are analyzed regularly without manual intervention.
Automating User Account Management
Managing user accounts is another area where automation can significantly enhance efficiency and accuracy.
Script to Create New User Accounts: powershell code
Import-Csv -Path "C:\NewUsers.csv" | ForEach-Object { New-LocalUser -Name $_.Username -Password (ConvertTo-SecureString $_.Password -AsPlainText -Force) -FullName $_.FullName -Description $_.Description Add-LocalGroupMember -Group "Users" -Member $_.Username }
Benefits:
Automates the creation of multiple user accounts from a CSV file.
Ensures consistency in account setup and reduces the time required for user provisioning.
Script to Disable Inactive User Accounts: powershell code
$threshold = (Get-Date).AddDays(-90) Get-LocalUser | Where-Object { $_.LastLogon -lt $threshold } | ForEach-Object { Disable-LocalUser -Name $_.Name }
Benefits:
Automatically identifies and disables user accounts that have been inactive for a specified period.
Enhances security by minimizing the risk of unused accounts being exploited.
Automating System Monitoring
Regular system monitoring is essential for maintaining security and performance. PowerShell scripts can automate this process, providing timely alerts and reports.
Script to Monitor Disk Space: powershell code
$drives = Get-PSDrive -PSProvider FileSystem $drives | ForEach-Object { if ($_.Used -gt ($_.Used + $_.Free) * 0.9) { Send-MailMessage -From "admin@example.com" -To "admin@example.com" -Subject "Disk Space Alert" -Body "Drive $($_.Name) is over 90% full." -SmtpServer "smtp.example.com" } }
Benefits:
Automatically monitors disk space and sends alerts if usage exceeds a threshold.
Helps in proactive management of disk space, preventing outages due to full disks.
Script to Check for Pending Updates: powershell code
$updates = Get-WindowsUpdate -KBArticleID KB1234567 if ($updates -ne $null) { Send-MailMessage -From "admin@example.com" -To "admin@example.com" -Subject "Pending Updates" -Body "There are pending updates on the system." -SmtpServer "smtp.example.com" }
Benefits:
Automates the check for pending system updates and sends notifications.
Ensures systems remain up-to-date with the latest security patches.
By automating these tasks with PowerShell, you can significantly enhance the efficiency and effectiveness of your cybersecurity operations. Automation not only saves time but also ensures that critical tasks are performed consistently and accurately. Next, we’ll discuss how to manage systems with PowerShell, further extending its capabilities in cybersecurity.
Managing Systems with PowerShell
PowerShell is a powerful tool for system administrators, offering extensive capabilities for managing Windows systems. It simplifies remote management, configuration changes, and updates, allowing for more efficient and consistent administration. Here’s an overview of how PowerShell can streamline various administrative tasks, complete with practical examples.
Remote Management
PowerShell provides robust features for managing systems remotely, making it easier to administer multiple machines from a single location.
Establishing a Remote Session:
Cmdlet: Enter-PSSession
Example: powershell code
Enter-PSSession -ComputerName Server01 -Credential (Get-Credential)
This command starts a remote session on Server01
using specified credentials.
Executing Commands Remotely:
Cmdlet: Invoke-Command
Example: powershell code
Invoke-Command -ComputerName Server01, Server02 -ScriptBlock { Get-Process }
This command runs Get-Process
on Server01
and Server02
simultaneously.
Managing Remote Sessions:
Cmdlet: New-PSSession
, Get-PSSession
, Remove-PSSession
Example: powershell code
$session = New-PSSession -ComputerName Server01 Invoke-Command -Session $session -ScriptBlock { Get-Service } Remove-PSSession -Session $session
This sequence of commands creates a remote session, executes a command, and then closes the session.
Configuration Changes
PowerShell simplifies making configuration changes across multiple systems, ensuring consistency and reducing manual effort.
Changing Network Configuration:
Cmdlet: Set-NetIPAddress
Example: powershell code
Set-NetIPAddress -InterfaceAlias "Ethernet" -IPAddress "192.168.1.100" -PrefixLength 24 -DefaultGateway "192.168.1.1"
This command sets a static IP address on the Ethernet interface.
Modifying System Settings:
Cmdlet: Set-ItemProperty
Example: powershell code
Set-ItemProperty -Path "HKLM:\System\CurrentControlSet\Control\Terminal Server" -Name "fDenyTSConnections" -Value 0
This command enables Remote Desktop connections by modifying a registry setting.
Managing Services:
Cmdlet: Start-Service
, Stop-Service
, Restart-Service
Example:powershell code
Stop-Service -Name "Spooler" Start-Service -Name "Spooler"
These commands stop and start the Print Spooler service.
System Updates
PowerShell can be used to manage system updates, ensuring systems remain secure and up-to-date.
Checking for Updates:
Cmdlet: Get-WindowsUpdate
(requires PSWindowsUpdate module)
Example: powershell code
Install-Module -Name PSWindowsUpdate -Force Get-WindowsUpdate
This command lists available updates.
Installing Updates:
Cmdlet: Install-WindowsUpdate
(requires PSWindowsUpdate module)
Example: powershell code
Get-WindowsUpdate -AcceptAll -Install -AutoReboot
This command installs all available updates and automatically reboots the system if necessary.
Scheduling Update Checks:
Cmdlet: New-ScheduledTaskTrigger
, Register-ScheduledTask
Example: powershell code
$trigger = New-ScheduledTaskTrigger -Daily -At 3AM Register-ScheduledTask -Action (New-ScheduledTaskAction -Execute "powershell.exe" -Argument "Get-WindowsUpdate -Install -AutoReboot") -Trigger $trigger -TaskName "Daily Windows Update"
This command schedules a daily task to check for and install updates at 3 AM.
Streamlining Administrative Tasks
PowerShell can automate and streamline a wide range of administrative tasks, making system management more efficient.
User Account Management:
Cmdlet: New-LocalUser
, Add-LocalGroupMember
Example: powershell code
New-LocalUser -Name "JohnDoe" -Password (ConvertTo-SecureString "P@ssw0rd!" -AsPlainText -Force) -FullName "John Doe" -Description "Standard User" Add-LocalGroupMember -Group "Users" -Member "JohnDoe"
This script creates a new local user and adds them to the Users group.
Disk Management:
Cmdlet: Get-PSDrive
, New-PSDrive
Example: powershell code
Get-PSDrive -PSProvider FileSystem New-PSDrive -Name "Z" -PSProvider FileSystem -Root "\\Server\Share"
These commands list all file system drives and create a new mapped network drive.
System Monitoring:
Cmdlet: Get-Counter
Example: powershell code
Get-Counter -Counter "\Processor(_Total)\% Processor Time" -SampleInterval 5 -MaxSamples 10
This command monitors CPU usage over a period.
By leveraging PowerShell for system management, administrators can automate routine tasks, ensure consistency across systems, and enhance overall operational efficiency. Next, we’ll explore how PowerShell can specifically enhance security operations, further extending its utility in cybersecurity.
Enhancing Security Operations with PowerShell
PowerShell’s flexibility and extensive cmdlet library make it an invaluable tool for enhancing security operations. It can be used for incident response, threat hunting, and vulnerability assessment, providing powerful scripting capabilities to automate and streamline these processes. Here, we discuss advanced uses of PowerShell in cybersecurity and provide examples of scripts that can help detect and mitigate threats.
Incident Response
PowerShell can be instrumental in incident response, allowing you to quickly gather information and take action when a security incident occurs.
Collecting System Information:
Cmdlet: Get-ComputerInfo
Example: powershell code
$systemInfo = Get-ComputerInfo $systemInfo | Export-Csv -Path "C:\IncidentResponse\SystemInfo.csv" -NoTypeInformation
This script collects detailed system information and exports it to a CSV file for analysis.
Analyzing Event Logs:
Cmdlet: Get-WinEvent
Example: powershell code
$events = Get-WinEvent -LogName Security -FilterHashtable @{Id=4625;StartTime=(Get-Date).AddDays(-1)} $events | Select-Object TimeCreated, Id, LevelDisplayName, Message | Export-Csv -Path "C:\IncidentResponse\FailedLogons.csv" -NoTypeInformation
This script retrieves failed logon attempts from the Security event log over the past day and exports them for further investigation.
Isolating a Compromised System:
Cmdlet: Set-NetAdapter
Example: powershell code
Get-NetAdapter | Where-Object { $_.Status -eq "Up" } | Set-NetAdapter -AdminStatus Down
This script disables all network adapters on a compromised system to isolate it from the network.
Threat Hunting
PowerShell can be used to proactively hunt for threats within your network by analyzing logs, network traffic, and system behavior.
Detecting Suspicious Processes:
Cmdlet: Get-Process
Example: powershell code
$suspiciousProcesses = Get-Process | Where-Object { $_.Name -eq "powershell" -and $_.StartTime -lt (Get-Date).AddMinutes(-30) } $suspiciousProcesses | Select-Object Name, Id, StartTime, CPU | Export-Csv -Path "C:\ThreatHunting\SuspiciousProcesses.csv" -NoTypeInformation
This script identifies PowerShell processes that have been running for more than 30 minutes, which can be indicative of malicious activity.
Monitoring Network Connections:
Cmdlet: Get-NetTCPConnection
Example: powershell code
$connections = Get-NetTCPConnection | Where-Object { $_.RemoteAddress -like "192.168.1.*" -and $_.State -eq "Established" } $connections | Select-Object LocalAddress, LocalPort, RemoteAddress, RemotePort, State | Export-Csv -Path "C:\ThreatHunting\NetworkConnections.csv" -NoTypeInformation
This script monitors network connections to and from a specified IP range and logs established connections.
Vulnerability Assessment
PowerShell can automate the process of checking for system vulnerabilities and ensuring compliance with security policies.
Checking for Missing Updates:
Cmdlet: Get-WindowsUpdate
(requires PSWindowsUpdate module)
Example: powershell code
Install-Module -Name PSWindowsUpdate -Force $missingUpdates = Get-WindowsUpdate -IsInstalled $false $missingUpdates | Select-Object Title, KBArticleID, Size, InstallDate | Export-Csv -Path "C:\VulnerabilityAssessment\MissingUpdates.csv" -NoTypeInformation
This script lists all missing updates on the system and exports the details to a CSV file.
Assessing Firewall Rules:
Cmdlet: Get-NetFirewallRule
Example: powershell code
$firewallRules = Get-NetFirewallRule | Where-Object { $_.Action -eq "Allow" } $firewallRules | Select-Object DisplayName, Enabled, Direction, LocalAddress, RemoteAddress, Protocol, Action | Export-Csv -Path "C:\VulnerabilityAssessment\FirewallRules.csv" -NoTypeInformation
This script retrieves all firewall rules that allow traffic and exports the details for review.
By leveraging PowerShell for these advanced cybersecurity tasks, you can significantly enhance your ability to detect, respond to, and mitigate security threats. These scripts provide a starting point for automating complex security operations, enabling you to focus on strategic activities that require human expertise. Next, we’ll summarize the key points covered and encourage further exploration of PowerShell in cybersecurity.
Embrace the Power of PowerShell in Cybersecurity
Throughout this article, we’ve highlighted the immense potential of PowerShell in the field of cybersecurity. By mastering PowerShell, you can significantly enhance your efficiency and effectiveness in managing systems, automating tasks, and responding to security threats.
Here’s a quick recap of what we’ve covered:
- Introduction to PowerShell: Understanding its role and benefits in cybersecurity.
- Basics and Syntax: Learning the fundamental elements and structure of PowerShell scripts.
- Essential Cmdlets: Exploring important cmdlets for system information, network configuration, and security management.
- Automating Tasks: Writing scripts to automate log analysis, user account management, and system monitoring.
- Managing Systems: Leveraging PowerShell for remote management, configuration changes, and system updates.
- Enhancing Security Operations: Using PowerShell for incident response, threat hunting, and vulnerability assessment.
PowerShell is more than just a scripting language—it’s a powerful tool that can transform your cybersecurity operations. By integrating PowerShell into your daily workflows, you can automate repetitive tasks, streamline system administration, and enhance your ability to detect and mitigate threats.
Resources for Further Learning
To continue your PowerShell journey, here are some valuable resources:
- Official Documentation: Explore the Microsoft PowerShell Documentation for comprehensive guides and references.
- Online Courses: Enroll in courses on platforms like Coursera, Udemy, and Pluralsight to deepen your understanding.
- Community Forums: Join forums such as Stack Overflow and the PowerShell.org community to engage with other professionals and learn from their experiences.
- Books: Read books like Learn Windows PowerShell in a Month of Lunches by Don Jones and Jeffrey Hicks, which offer practical, hands-on learning.
Engage with the Community
We encourage all members and visitors of the BugBustersUnited community to share their experiences with PowerShell. Whether you’re a beginner or an experienced user, your insights and stories are valuable:
- Share Your Usage: How are you using PowerShell in your cybersecurity tasks? What scripts have you found most helpful?
- Discuss the Goods and Bads: What successes have you achieved with PowerShell? What challenges have you faced, and how did you overcome them?
- Contribute to Improvement: Your feedback can help improve our collective understanding and application of PowerShell in cybersecurity.
By embracing PowerShell and continuously learning, you can elevate your cybersecurity skills and make a meaningful impact in your organization. Let’s harness the power of automation together and create a safer digital world. Happy scripting, BugBusters!