Swift in iOS Security: A Modern Approach to Mobile Threats
Utilizing Swift for Secure iOS Application Development and Analysis
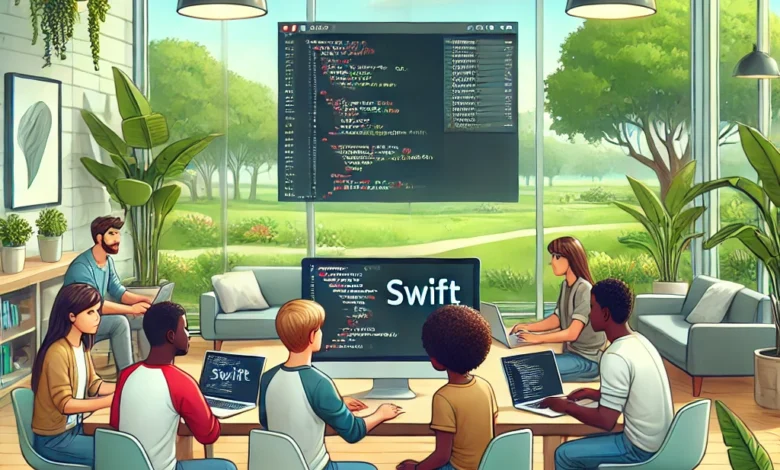
In today’s world, where our lives are deeply intertwined with mobile devices, iOS security has never been more critical. From online banking to personal communication, our iPhones and iPads hold some of our most sensitive information. This is why securing these devices—and the apps that run on them—is a top priority for cybersecurity professionals.
Enter Swift: Apple’s modern, powerful programming language designed specifically for iOS development. But Swift isn’t just any programming language; it’s a game-changer when it comes to building secure, resilient apps. With its clean, expressive syntax and robust safety features, Swift helps developers write code that’s not only efficient but also far less prone to the kinds of vulnerabilities that attackers love to exploit.
For security professionals and aspiring bug bounty hunters, mastering Swift is like adding a secret weapon to your arsenal. Whether you’re developing secure apps from scratch or analyzing existing ones for potential threats, Swift gives you the tools to do it better, faster, and with greater confidence.
In this article, we’ll dive into why Swift is becoming the go-to language for iOS security. We’ll explore its unique features, show you how it can enhance your security efforts, and help you understand why getting comfortable with Swift is a must for anyone serious about protecting iOS apps and users. So, buckle up, BugBusters—let’s see why Swift is making waves in the world of mobile security!
Swift’s Safety Features: Built-In Security for Developers
When it comes to secure iOS app development, Swift stands out, not just because it’s modern and easy to use, but because it’s built with safety at its core. This is no small feat—security vulnerabilities often arise from simple coding mistakes, and Swift’s design helps prevent those pitfalls right from the start.
Optional: Guarding Against Unexpected Failures
One of Swift’s most lauded features is its use of optionals. In many programming languages, accessing a variable that has no value (a null pointer) can lead to crashes or, worse, security vulnerabilities. Swift handles this by making it clear when a variable might not have a value, forcing developers to write code that safely handles these scenarios. For example:
var username: String? // This is an optional
username = "BugBuster" // Assign a value
By explicitly declaring variables as optionals, Swift ensures that developers account for every possible outcome, reducing the likelihood of null pointer dereferencing—a common source of bugs and security issues.
Strong Typing: Ensuring Code Reliability
Swift’s strong typing system is another line of defense. Unlike loosely typed languages where variables can change type unexpectedly, Swift requires developers to define the type of each variable. This rigidity helps catch errors early in the development process, preventing unexpected behaviors that could be exploited by attackers.
let age: Int = 25
let name: String = "BugBuster"
In this code, the type of each variable is explicitly defined, meaning the compiler can catch any type-related errors before the app even runs. This reduces the risk of type-related vulnerabilities, making your iOS apps more secure.
Memory Management: Preventing Overflows
Memory safety is critical in preventing issues like buffer overflows, which can lead to serious vulnerabilities. Swift manages memory automatically, helping to avoid the common pitfalls associated with manual memory management in languages like C or C++. With Swift, memory is allocated and deallocated safely, reducing the chances of security flaws related to memory misuse.
For example, when using collections like arrays, Swift’s built-in bounds checking ensures you don’t accidentally read or write outside the bounds of the array:
let numbers = [1, 2, 3, 4]
print(numbers[4]) // Swift prevents out-of-bounds access
If you attempt to access an index that doesn’t exist, Swift will catch it at runtime, preventing potential crashes or exploitable conditions.
Putting It All Together
These safety features—optional, strong typing, and automatic memory management—are integral to Swift’s design. They help developers write secure code by default, making it harder for vulnerabilities to creep in. If you’re a security researcher or a developer looking to build robust iOS applications, leveraging Swift’s built-in safety features is a no-brainer.
If you’re new to Swift or want to dive deeper, you can start by downloading Xcode, Apple’s integrated development environment (IDE), which includes everything you need to get started with Swift. Check out the official Swift documentation for comprehensive guides and tutorials, and explore communities like Swift Forums to connect with other developers.
By embracing Swift’s safety features, you’re not just writing better code—you’re building a more secure digital future.
Developing Secure iOS Applications with Swift
When it comes to mobile security, the stakes are high. Users trust iOS apps to handle their data securely, and any breach can lead to significant consequences. As a developer, your goal is to write code that not only functions well but also protects against potential security threats. Swift, with its robust features, can be your ally in this endeavor. Let’s explore how to use Swift to develop secure iOS applications that can stand up to modern threats.
Input Validation: The First Line of Defense
One of the most critical aspects of secure coding is input validation. By ensuring that any data your app processes is valid and expected, you can prevent a wide range of attacks, including SQL injection and cross-site scripting (XSS).
In Swift, you can use functions like filter
, map
, and reduce
to sanitize inputs effectively. For example, consider an app that requires user input to search a database:
func sanitizeInput(_ input: String) -> String {
let allowedCharacters = CharacterSet.alphanumerics
return input.filter { allowedCharacters.contains($0.unicodeScalars.first!) }
}
let userInput = "DROP TABLE Users;--"
let safeInput = sanitizeInput(userInput)
In this snippet, sanitizeInput
removes any characters from the user’s input that aren’t alphanumeric, effectively neutralizing potentially harmful inputs. By ensuring that only valid data is processed, you reduce the risk of SQL injection and other input-related attacks.
Using Prepared Statements to Prevent SQL Injection
While input validation is crucial, combining it with prepared statements provides an additional layer of security against SQL injection. Prepared statements ensure that SQL code and data are kept separate, making it impossible for an attacker to manipulate SQL queries through input.
Here’s how you can use prepared statements with Swift’s SQLite
library:
import SQLite3
let db: OpaquePointer?
if sqlite3_open("myDatabase.db", &db) == SQLITE_OK {
let queryString = "SELECT * FROM Users WHERE username = ?"
var stmt: OpaquePointer?
if sqlite3_prepare_v2(db, queryString, -1, &stmt, nil) == SQLITE_OK {
sqlite3_bind_text(stmt, 1, safeInput, -1, nil)
while sqlite3_step(stmt) == SQLITE_ROW {
let username = String(cString: sqlite3_column_text(stmt, 1))
print("User: \(username)")
}
}
sqlite3_finalize(stmt)
}
This code snippet demonstrates how to use a prepared statement to execute a SQL query securely. By binding the user input as a parameter, you prevent it from being executed as SQL code, thereby protecting against SQL injection attacks.
Encryption: Protecting Sensitive Data
Data encryption is another cornerstone of secure app development. Whether you’re storing data on the device or transmitting it over a network, encrypting sensitive information ensures that even if it’s intercepted or accessed, it remains unreadable.
Swift makes it easy to implement encryption using the CryptoKit
framework. Here’s an example of encrypting a piece of data:
import CryptoKit
let password = "SuperSecretPassword123!"
let passwordData = Data(password.utf8)
let key = SymmetricKey(size: .bits256)
let sealedBox = try! ChaChaPoly.seal(passwordData, using: key)
let ciphertext = sealedBox.ciphertext
print("Encrypted password: \(ciphertext)")
In this example, ChaChaPoly
is used to encrypt a password. The encrypted data (ciphertext
) is what you would store or transmit, ensuring that the original password is not exposed.
Error Handling: Avoiding Information Leakage
When writing secure iOS apps, it’s important to handle errors in a way that doesn’t expose sensitive information. Swift’s error handling is powerful and flexible, but if misused, it can unintentionally reveal details that attackers can exploit.
For instance, consider a login function that might expose whether a username or password was incorrect:
enum LoginError: Error {
case invalidCredentials
case accountLocked
}
func login(username: String, password: String) throws {
guard isValidUser(username: username, password: password) else {
throw LoginError.invalidCredentials
}
if isAccountLocked(username: username) {
throw LoginError.accountLocked
}
// Proceed with login
}
Here, it’s tempting to provide detailed error messages. However, it’s safer to return a generic message:
do {
try login(username: "testUser", password: "wrongPassword")
} catch {
print("Login failed. Please try again.")
}
This way, attackers don’t gain any additional information that could aid in brute-force or enumeration attacks.
Putting It All Together
Developing secure iOS applications requires a combination of good coding practices and an understanding of potential threats. Swift’s features—like optionals, strong typing, and modern libraries—give you the tools you need to write secure code that protects user data and resists attacks.
Start by integrating these practices into your development workflow. Regularly review your code for potential vulnerabilities, and make sure you’re up to date with the latest security standards. By leveraging Swift’s capabilities, you’re not just building apps—you’re creating a safer mobile experience for users everywhere.
Reverse Engineering iOS Apps: Swift in Action
Reverse engineering is a critical process in the field of cybersecurity, especially when it comes to iOS apps. By dissecting and analyzing the internal workings of an application, security researchers can uncover vulnerabilities that might not be apparent during standard testing. Swift, Apple’s modern programming language, is at the core of many iOS apps today. Understanding Swift’s syntax and structure can greatly enhance your ability to perform effective reverse engineering on iOS applications, ultimately leading to more thorough security analyses.
Understanding Reverse Engineering in iOS Security
Reverse engineering involves taking an application apart to understand how it works internally. For iOS apps, this typically means decompiling the application’s binary, examining its code, and analyzing its behavior to identify potential vulnerabilities. This process is essential for uncovering issues like insecure data storage, improper encryption, and other security flaws that could be exploited by attackers.
In the context of iOS security, reverse engineering is often used to:
- Analyze the app’s logic and detect hidden functionalities or backdoors.
- Understand how sensitive data is handled within the app.
- Identify weaknesses in encryption and data protection mechanisms.
Tools and Techniques for Decompiling Swift-Based iOS Apps
Reverse engineering Swift-based iOS applications requires specialized tools and techniques, given Swift’s modern and often complex syntax. Below are some commonly used tools and methods:
- Class-Dump and Hopper Disassembler:
- Class-Dump: This tool is used to extract class and method information from Objective-C and Swift binaries. It generates headers that can give you insights into the app’s structure.
- Hopper Disassembler: A powerful disassembler that helps you visualize and analyze the assembly code of a Swift-based app. Hopper supports Swift, allowing you to view the app’s functions, strings, and control flow.
class-dump -H -o ~/Desktop/Headers AppName.app/AppName
This command extracts the app’s headers, providing a starting point for understanding the app’s architecture.
Ghidra:
- Developed by the NSA, Ghidra is an open-source reverse engineering tool that can decompile iOS binaries. It supports Swift and is excellent for understanding higher-level constructs and how they map to the binary code.
Example: After loading the binary into Ghidra, you can navigate through the code and identify key functions and data structures that might be of interest in your analysis.
Frida and Radare2:
- Frida: A dynamic instrumentation toolkit that allows you to hook into a running iOS app. You can inject scripts to interact with the app’s code in real time, making it easier to understand how specific functions work and how data is manipulated.
- Radare2: An advanced command-line tool that provides deep insights into the binary code, offering features like debugging, disassembling, and hex editing.
Example:
frida -U -n AppName -l script.js
- This command attaches Frida to a running iOS app, allowing you to manipulate and observe its behavior directly.
Interpreting Swift’s Syntax and Structure
Swift’s syntax is designed to be safe and easy to use, but it also introduces complexities that can make reverse engineering challenging. When analyzing a Swift-based iOS app, understanding the following key aspects is crucial:
Optional: Swift uses optionals to handle the absence of a value. When reverse engineering, pay attention to how optionals are unwrapped, as improper handling could lead to vulnerabilities.Example:
guard let unwrappedValue = optionalValue else {
// Handle error
return
}
// Use unwrappedValue safely
Identifying areas where optionals are forcefully unwrapped (!
) can highlight potential crash points or error-handling flaws.
Memory Management: Swift’s memory management, while automatic, still requires careful examination during reverse engineering. Look for potential memory leaks or unsafe pointer manipulations, which could lead to exploitable vulnerabilities.
Method Swizzling: In Swift, method swizzling can be used to change the implementation of existing methods at runtime. This technique is often used to modify app behavior dynamically. Understanding how and where swizzling occurs can reveal hidden or unexpected functionalities.
Example:
import ObjectiveC
extension UIViewController {
static let swizzleViewDidLoad: Void = {
let originalSelector = #selector(viewDidLoad)
let swizzledSelector = #selector(swizzled_viewDidLoad)
let originalMethod = class_getInstanceMethod(UIViewController.self, originalSelector)
let swizzledMethod = class_getInstanceMethod(UIViewController.self, swizzledSelector)
method_exchangeImplementations(originalMethod!, swizzledMethod!)
}()
@objc func swizzled_viewDidLoad() {
// Call the original method
swizzled_viewDidLoad()
print("ViewDidLoad swizzled!")
}
}
Recognizing such patterns during reverse engineering can help you uncover how an app might change its behavior under certain conditions.
Applying Reverse Engineering Insights to Security Analysis
By thoroughly understanding Swift’s structure and using the right tools, you can uncover vulnerabilities that might otherwise go unnoticed. This knowledge allows you to:
- Identify Weak Points: Pinpoint areas in the code where the app is most vulnerable to attacks, such as improper handling of sensitive data or weak encryption methods.
- Enhance App Security: Provide feedback to developers on potential security flaws, contributing to the development of more secure iOS applications.
- Contribute to Bug Bounty Programs: Use your reverse engineering skills to discover critical vulnerabilities in apps, which can lead to rewards in bug bounty programs.
Swift as a Gateway to Advanced iOS Security Analysis
Reverse engineering Swift-based iOS apps is a complex but rewarding process. With the right tools and a deep understanding of Swift’s syntax and structure, you can uncover critical vulnerabilities and contribute to more secure mobile applications. Whether you’re a security researcher or an aspiring bug bounty hunter, mastering the art of reverse engineering in Swift can significantly enhance your security analysis capabilities, making you a valuable asset in the ongoing battle against mobile threats.
Using Swift for Mobile Threat Analysis
In the rapidly evolving landscape of mobile security, Swift has proven to be more than just a language for building iOS apps—it’s also a powerful tool for analyzing and mitigating mobile threats. As iOS continues to dominate the mobile market, the security of apps on this platform is of paramount importance. Swift’s modern language features, combined with its seamless integration with iOS, make it an ideal choice for security researchers focused on mobile threat analysis.
Analyzing Mobile Threats with Swift
Mobile threats like malware, phishing attacks, and unauthorized data access have become increasingly sophisticated. Security researchers utilize Swift to dissect these threats, uncovering their mechanics and developing countermeasures.
- Swift for Malware Analysis: Swift is used to create tools that analyze the behavior of suspicious apps. By leveraging Swift’s ability to interact with iOS APIs, researchers can monitor how an app interacts with the system, including network requests, file system changes, and more. Example: Imagine a Swift-based tool that monitors network traffic within an app. This tool can be designed to detect unusual patterns, such as frequent requests to a known malicious domain, which may indicate a phishing attempt or a data exfiltration scheme.
import Foundation
import Network
class NetworkMonitor {
private let monitor: NWPathMonitor
private let queue = DispatchQueue.global()
init() {
monitor = NWPathMonitor()
}
func startMonitoring() {
monitor.pathUpdateHandler = { path in
if path.status == .satisfied {
print("We're connected!")
} else {
print("No connection.")
}
path.availableInterfaces.forEach { interface in
print("Available interface: \(interface.debugDescription)")
}
if path.usesInterfaceType(.wifi) {
print("Using WiFi")
} else if path.usesInterfaceType(.cellular) {
print("Using Cellular")
}
}
monitor.start(queue: queue)
}
func stopMonitoring() {
monitor.cancel()
}
}
let networkMonitor = NetworkMonitor()
networkMonitor.startMonitoring()
This Swift code snippet shows how you can use the Network
framework to monitor network changes and interfaces. Expanding on this, you can log connections, analyze destination addresses, and flag any traffic that matches known threat indicators.
Detecting Phishing Attacks with Swift: Phishing attacks often target mobile users, exploiting their trust in well-designed apps. Security researchers can use Swift to build tools that analyze URLs within iOS apps, flagging those that match patterns commonly associated with phishing attempts.
Example: A Swift tool could be built to scan URLs in an app for known phishing domains or to look for suspicious patterns, such as domains that mimic legitimate websites.
let phishingDomains = ["fakebank.com", "phishy.com"]
let urlToCheck = URL(string: "https://fakebank.com/login")!
if phishingDomains.contains(where: urlToCheck.host?.contains ?? { _ in false }) {
print("Warning: Potential phishing URL detected!")
} else {
print("URL is safe.")
}
- This simple Swift snippet checks if a URL belongs to a list of known phishing domains. Expanding on this, you could integrate it into a real-time analysis tool that checks URLs as users interact with them, providing instant feedback and protection.
Building Tools to Respond to Mobile Threats
Swift’s strong typing, safety features, and powerful integration with iOS make it ideal for developing tools that not only detect but also respond to mobile threats. By leveraging Swift’s capabilities, security professionals can automate responses to threats, such as blocking malicious URLs, quarantining suspicious files, or notifying users of potential risks.
- Automated Response Tools: Using Swift, you can create tools that automatically respond to detected threats. For example, a tool could be built to automatically close a compromised app and alert the user if it detects malicious behavior.Example: Suppose a Swift-based monitoring tool detects that an app is trying to access a user’s contacts without permission. The tool could immediately revoke that permission and alert the user.
import Contacts
let store = CNContactStore()
func revokeContactsAccess(for appBundleID: String) {
// Logic to revoke contacts access
print("Contacts access revoked for \(appBundleID)")
}
revokeContactsAccess(for: "com.suspicious.app")
- In this example, the code outlines a simple function that, when integrated with broader monitoring tools, could revoke app permissions dynamically based on detected malicious behavior.
- Case Studies: Swift-Based Tools in ActionCase Study 1: PhishDetector
- A Swift-based tool designed to integrate with iOS apps to detect and block phishing attempts in real-time. PhishDetector analyzes URLs before they load, comparing them against a constantly updated list of known phishing domains. When a match is found, it prevents the connection and alerts the user.
- This tool was developed in Swift to monitor app behavior, focusing on unauthorized data access. iOSGuard tracks apps’ attempts to access sensitive user data, such as contacts or location, and blocks any attempts that don’t align with the app’s expected behavior profile. This proactive security measure has been pivotal in protecting users from apps that bypass App Store scrutiny.
Swift’s Role in Proactive Security Measures
Using Swift, security researchers, and developers can stay ahead of threats, ensuring that iOS apps remain secure against emerging risks. Swift’s modern, robust language features enable the development of sophisticated tools that not only detect threats but also respond to them in real-time, minimizing potential damage.
Swift isn’t just a tool for app development—it’s an essential component of modern iOS security strategy. From building automated threat detection systems to developing tools that secure user data, Swift provides the capabilities needed to safeguard mobile apps in an increasingly hostile cyber landscape.
Swift as the Future of iOS Threat Analysis
Incorporating Swift into your mobile threat analysis toolkit can significantly enhance your ability to detect and mitigate security threats on iOS. With its strong safety features, seamless iOS integration, and powerful programming capabilities, Swift is positioned to become a cornerstone in the future of mobile cybersecurity. Whether you’re developing new apps or securing existing ones, mastering Swift for security purposes is a strategic move that will equip you to tackle the challenges of mobile threats head-on.
Swift vs. Objective-C: The Security Perspective
When it comes to developing secure iOS applications, the choice between Swift and Objective-C is crucial. Both languages have been instrumental in the evolution of Apple’s ecosystem, but Swift, as a more modern language, offers several security advantages that make it a strong contender for developers focused on creating secure applications.
Memory Management: Swift’s Safe Handling vs. Objective-C’s Vulnerabilities
One of the most significant differences between Swift and Objective-C is how they handle memory management, a critical factor in application security. Memory management issues are a common source of security vulnerabilities, particularly in languages that rely on manual memory management, such as Objective-C.
Objective-C:
In Objective-C, developers must manually manage memory using concepts like retain
, release
, and autorelease
. While powerful, this manual management is prone to human error, leading to vulnerabilities like memory leaks and buffer overflows. Attackers can exploit these errors to execute arbitrary code or cause a program to crash, both of which compromise the security of the application.
Swift:
On the other hand, Swift introduced Automatic Reference Counting (ARC), which automates memory management. ARC automatically keeps track of memory allocation and deallocation, significantly reducing the chances of memory leaks or overflows. This automated approach not only simplifies the development process but also minimizes the risk of security vulnerabilities associated with improper memory management.
Example: Consider a scenario where a developer is managing an array of sensitive data, such as user passwords. In Objective-C, manual memory management could easily lead to a situation where a buffer overflow occurs, potentially exposing this sensitive information.
In Swift, ARC automatically handles memory allocation and deallocation, ensuring that sensitive data is managed securely, with less risk of accidental exposure due to human error.
class SecureDataHandler {
var passwords: [String] = []
func addPassword(_ password: String) {
passwords.append(password)
}
deinit {
passwords.removeAll() // ARC handles memory cleanup securely
}
}
In this Swift example, ARC ensures that when the SecureDataHandler
an instance is deallocated, the memory used by the password array is properly released, preventing any potential memory management issues that could lead to vulnerabilities.
Error Handling: Swift’s Safety vs. Objective-C’s Flexibility
Another area where Swift shines is its approach to error handling. In Objective-C, error handling is done through the use of NSError objects and return codes, which can be prone to misuse or oversight. This can result in unhandled errors, leading to crashes or unexpected behavior—both of which are security risks.
Objective-C: Objective-C relies heavily on conventions, where developers must remember to check and handle errors correctly. This approach, while flexible, leaves room for oversight, where a missed error check can lead to security issues.
Swift: Swift introduces a robust error-handling mechanism using do-catch
blocks, try
, and throw
keywords. This structured approach makes it clear when errors need to be handled, reducing the likelihood of unhandled exceptions or ignored errors. By forcing developers to address potential errors directly, Swift helps ensure that the application’s error-handling logic is both thorough and secure.
Example: Imagine a situation where an app needs to read data from a file that may not exist. In Objective-C, if the error handling isn’t implemented correctly, the app might crash or, worse, expose sensitive data by mishandling the error.
In Swift, the do-catch
structure makes it explicit that errors must be handled, improving the overall security of the application.
func readFileContents(path: String) throws -> String {
guard let fileContent = try? String(contentsOfFile: path) else {
throw FileError.fileNotFound
}
return fileContent
}
do {
let content = try readFileContents(path: "user_data.txt")
print(content)
} catch {
print("An error occurred: \(error)")
}
This Swift example clearly outlines how errors are managed. If the file isn’t found, the error is caught, preventing the app from crashing and allowing for secure error handling.
Null Safety: Eliminating Null Pointer Dereferencing
Null pointer dereferencing is a notorious source of bugs and security vulnerabilities in many programming languages, including Objective-C. When a program attempts to access or modify data through a null pointer, it can lead to crashes, unpredictable behavior, or even security breaches.
Objective-C:
In Objective-C, dealing with nil
(the equivalent of null) pointers requires careful checks throughout the code. If a developer misses a nil
check, it can result in a null pointer dereference, which can be exploited by attackers to execute malicious code.
Swift:
Swift’s approach to null safety is a game-changer in this regard. Swift uses optionals, a language feature that explicitly requires developers to handle the possibility of nil
values. This means that a variable must be explicitly unwrapped or handled before it can be used, greatly reducing the risk of null pointer dereferencing.
Example: Consider a scenario where an application fetches user data from a server. If the data is missing or corrupt, this could lead to a nil
the value being processed.
In Swift, the use of optionals forces the developer to handle this case explicitly, thereby improving the application’s security posture.
var userData: String?
func fetchUserData() {
guard let data = userData else {
print("No data available.")
return
}
print("User data: \(data)")
}
fetchUserData()
In this example, Swift’s use of options (String?) ensures that the userData a variable is checked for nil
before it’s used, preventing any null pointer issues.
When to Choose Swift Over Objective-C
From a security perspective, Swift is often the preferred choice for modern iOS development. Its strong typing, memory management, and robust error handling make it a safer language that minimizes common vulnerabilities found in Objective-C. However, Objective-C might still be relevant in legacy projects or when working with older codebases that heavily rely on it.
Security-focused Developers Should Choose Swift When:
- Starting a new iOS project with security as a priority.
- Refactoring existing code to enhance security and reliability.
- Developing apps that handle sensitive user data where null safety and memory management are crucial.
Objective-C May Still Be Relevant When:
- Working on legacy applications with significant Objective-C codebases.
- Integrating with older libraries or frameworks written in Objective-C.
Swift as the Secure Choice
In conclusion, while both Swift and Objective-C have their places in iOS development, Swift clearly offers superior security features that help developers build more secure applications. Its modern design addresses many of the pitfalls that can lead to vulnerabilities in Objective-C, making it the language of choice for developers focused on security. By leveraging Swift’s robust safety features, iOS developers can significantly reduce the risk of security breaches and ensure a safer experience for their users.
Getting Started with Swift for iOS Security
If you want to dive into iOS security, mastering Swift is a powerful way to start. With Swift’s growing prominence in iOS development, becoming proficient in this language will enable you to build secure apps, analyze mobile threats, and contribute meaningfully to the cybersecurity community. Here’s a guide to help you get started on this journey.
Choosing the Right Learning Resources
To get a solid foundation in Swift, especially with a focus on iOS security, it’s essential to pick the right resources. Below are some top recommendations:
- Online Courses:
- Udemy’s iOS 15 & Swift 5: The Complete iOS App Development Bootcamp: This course, while primarily focused on app development, covers key Swift concepts that are crucial for building secure applications. It’s a comprehensive starting point for beginners.
- Coursera’s iOS App Development with Swift Specialization: This is a beginner-friendly course that introduces Swift from scratch and is particularly useful for understanding how Swift’s safety features can be applied to secure iOS development.
- Books:
- Swift Programming: The Big Nerd Ranch Guide: This book is perfect for developers who prefer a hands-on approach. It covers Swift in-depth and provides practical examples that can be adapted for security purposes.
- iOS Application Security: The Definitive Guide for Hackers and Developers by David Thiel: This book focuses specifically on security in iOS development, making it a valuable resource for those looking to understand the intersection of Swift and security.
- Tutorials and Documentation:
- Apple’s Swift Documentation: Apple’s official documentation is always a great reference. It provides in-depth explanations of Swift’s features, with plenty of examples.
- Swift Programming Tutorial: Vandad Nahavandipoor
- offers a wide range of tutorials on Swift, including security-focused projects that help you practically apply what you’ve learned.
Starting with Security-Focused Projects
One of the best ways to learn Swift for iOS security is by building projects focusing on secure coding practices. Here are some project ideas to get you started:
- Secure Password Manager: Build a simple iOS password manager that uses Swift’s encryption libraries to store and retrieve user credentials securely. This project will help you understand how to implement secure data storage and encryption in Swift.
- Authentication App: Create an app that uses biometrics (like Face ID or Touch ID) for authentication. This project will introduce you to working with sensitive user data and ensuring that your app meets the highest security standards.
- Network Security Tool: Develop a tool that monitors network traffic on an iOS device, identifying potential vulnerabilities or suspicious activity. This will give you hands-on experience with Swift’s networking capabilities and security features.
Example:
Imagine building a simple secure notes app using Swift. In this app, you can use Swift CryptoKit to encrypt notes before saving them to the device. This would involve understanding key management and secure data storage practices, which are essential skills in iOS security.
import CryptoKit
func encrypt(text: String, using key: SymmetricKey) -> Data? {
let data = Data(text.utf8)
let sealedBox = try? AES.GCM.seal(data, using: key)
return sealedBox?.combined
}
func decrypt(data: Data, using key: SymmetricKey) -> String? {
guard let box = try? AES.GCM.SealedBox(combined: data),
let decryptedData = try? AES.GCM.open(box, using: key) else {
return nil
}
return String(data: decryptedData, encoding: .utf8)
}
This basic example shows how you might handle encryption and decryption within an app, a critical component of building secure iOS applications.
Contributing to Open-Source iOS Security Projects
Another excellent way to deepen your Swift and security skills is to contribute to open-source projects. This not only gives you real-world experience, but it also helps you connect with other professionals in the field.
- OWASP’s iMAS (iOS Mobile Application Security) Project: This project is focused on developing open-source security controls for iOS applications. Contributing to iMAS can help you understand advanced security concepts and how they are implemented in real-world iOS apps.
- Mobile Security Framework (MobSF): MobSF is an open-source mobile security framework that helps automate the analysis of mobile applications. By contributing to this project, you can gain hands-on experience in reverse engineering and analyzing iOS apps.
Example:
If you decide to contribute to MobSF, you might start by improving the Swift codebase or adding new features that enhance the framework’s ability to detect vulnerabilities in Swift-based iOS applications. This not only strengthens your Swift skills but also gives you experience working with a widely-used security tool.
Experimenting and Building Confidence
Finally, it’s essential to experiment with Swift on your own. Start by creating small tools or applications that focus on security aspects. Over time, increase the complexity of your projects as you become more comfortable with the language.
- Daily Coding Challenges: Take on Swift coding challenges that focus on security scenarios. Websites like LeetCode and HackerRank often have problems that can be approached with a security mindset.
- Personal Security Projects: Try to replicate security features from popular apps or build tools that automate security testing for iOS applications.
Example:
Challenge yourself to build a simple app that securely handles API keys and user credentials. This project could involve securely storing these keys using Swift’s Keychain
APIs and ensuring that they are transmitted securely using HTTPS.
By engaging in these activities, you’ll not only improve your Swift programming skills but also deepen your understanding of iOS security, making you a more effective security professional or bug bounty hunter.
Taking the Next Step with Swift
Swift is a modern, powerful language that’s particularly well-suited for iOS security. By starting with the resources and projects outlined above, you’ll be well on your way to mastering Swift for secure iOS development. Whether you’re building secure apps, reverse-engineering existing ones, or developing tools to analyze mobile threats, Swift provides the features and flexibility needed to protect users in today’s mobile-driven world. So, dive in, start experimenting, and watch as your skills in Swift and iOS security grow.
Embrace Swift for a Secure iOS Future
As we wrap up our exploration of Swift in the context of iOS security, it’s clear that Swift offers a unique blend of modernity, safety, and performance that makes it an essential tool for any cybersecurity professional working with iOS applications. Swift’s safety features, such as optional and strong typing, reduce common vulnerabilities like buffer overflows and null pointer dereferencing, allowing you to write code that is not only efficient but also secure.
Swift’s modern syntax and built-in security features make it a natural choice for secure iOS app development. With Swift, you can build robust applications that are resistant to common security threats like SQL injection and cross-site scripting. Secure coding practices become more intuitive, enabling you to focus on protecting users from the ever-evolving landscape of mobile threats.
Example:
Consider a scenario where you’re tasked with developing a secure messaging app. Using Swift, you could implement end-to-end encryption with minimal risk of introducing security flaws due to Swift’s type safety and memory management features. The CryptoKit
framework in Swift makes it easier to handle encryption and decryption processes securely, while Swift’s concise syntax ensures that your code is both clean and maintainable.
Moreover, Swift proves invaluable in reverse engineering iOS applications. Its readability and structure make analyzing and understanding existing Swift-based apps more straightforward, which is crucial when you need to uncover vulnerabilities or assess the security posture of an application. Security researchers can use Swift’s robust features to develop tools that monitor and analyze iOS apps for potential security flaws.
Example:
Imagine developing a Swift-based tool that monitors API calls within an app to detect any suspicious activity. With Swift, you can leverage its interoperability with other security tools, enhancing your ability to perform detailed threat analysis and respond to emerging security issues quickly.
As you continue to explore Swift, you’ll find that its advantages extend beyond just development—it’s also a powerful language for analyzing and mitigating mobile threats. Whether you’re building a tool to detect malware in iOS apps or developing a secure authentication system, Swift equips you with the capabilities needed to stay ahead in the dynamic field of mobile security.
Example:
If you’re working in a cybersecurity team tasked with ensuring the security of a company’s iOS applications, using Swift allows you to integrate security features into the development pipeline seamlessly. From performing automated security tests to building real-time threat detection systems, Swift’s versatility ensures you can meet your security goals without compromising performance or user experience.
Final Thoughts:
The evolving landscape of mobile security demands tools and languages that are powerful and adaptable to the specific challenges of securing mobile applications. Swift is one such language—its combination of safety, performance, and ease of use makes it a compelling choice for anyone looking to excel in iOS security.
As you move forward, continue to explore Swift’s capabilities. Whether you’re a developer looking to build more secure apps or a security professional focused on threat analysis, integrating Swift into your toolset will significantly enhance your ability to protect iOS users. By mastering Swift, you’ll be better equipped to develop, analyze, and secure iOS applications, ensuring that you stay at the forefront of mobile cybersecurity.
Now is the time to embrace Swift and make it a cornerstone of your cybersecurity efforts. As you do, you’ll find that Swift simplifies the development process and empowers you to create more secure and resilient iOS applications, protecting users from the increasingly sophisticated threats that target mobile devices.